Java Reference
In-Depth Information
•A
throw
statement (p. 457) can throw any
Throwable
object.
• Exceptions are rethrown (p. 458) when a
catch
block, upon receiving an exception, decides ei-
ther that it cannot process that exception or that it can only partially process it. Rethrowing an
exception defers the exception handling (or perhaps a portion of it) to another
catch
block.
• When a rethrow occurs, the next enclosing
try
block detects the rethrown exception, and that
try
block's
catch
blocks attempt to handle it.
Section 11.7 Stack Unwinding and Obtaining Information from an Exception Object
• When an exception is thrown but not caught in a particular scope, the method-call stack is un-
wound, and an attempt is made to
catch
the exception in the next outer
try
statement.
• Class
Throwable
offers a
printStackTrace
method that prints the method-call stack. Often, this
is helpful in testing and debugging.
• Class
Throwable
also provides a
getStackTrace
method that obtains the same stack-trace infor-
mation that's printed by
printStackTrace
(p. 461).
• Class
Throwable
's
getMessage
method (p. 461) returns the descriptive string stored in an excep-
tion.
•Method
getStackTrace
(p. 461) obtains the stack-trace information as an array of
StackTrace-
Element
objects. Each
StackTraceElement
represents one method call on the method-call stack.
•
StackTraceElement
methods (p. 461)
getClassName
,
getFileName
,
getLineNumber
and
get-
MethodName
get the class name, filename, line number and method name, respectively.
Section 11.8 Chained Exceptions
• Chained exceptions (p. 462) enable an exception object to maintain the complete stack-trace in-
formation, including information about previous exceptions that caused the current exception.
Section 11.9 Declaring New Exception Types
• A new exception class must extend an existing exception class to ensure that the class can be used
with the exception-handling mechanism.
Section 11.10 Preconditions and Postconditions
• A method's precondition (p. 465) must be true when the method is invoked.
• A method's postcondition (p. 465) is true after the method successfully returns.
• When designing your own methods, you should state the preconditions and postconditions in a
comment before the method declaration.
Section 11.11 Assertions
• Assertions (p. 465) help catch potential bugs and identify possible logic errors.
•The
assert
statement (p. 466) allows for validating assertions programmatically.
• To enable assertions at runtime, use the
-ea
switch when running the
java
command.
Section 11.12
try
-with-Resources: Automatic Resource Deallocation
•The
try
-with-resources statement (p. 467) simplifies writing code in which you obtain a resource,
use it in a
try
block and release the resource in a corresponding
finally
block. Instead, you allocate
the resource in the parentheses following the
try
keyword and use the resource in the
try
block;
then the statement implicitly calls the resource's
close
method at the end of the
try
block.
• Each resource must be an object of a class that implements the
AutoCloseable
interface
(p. 467)—such a class has a
close
method.
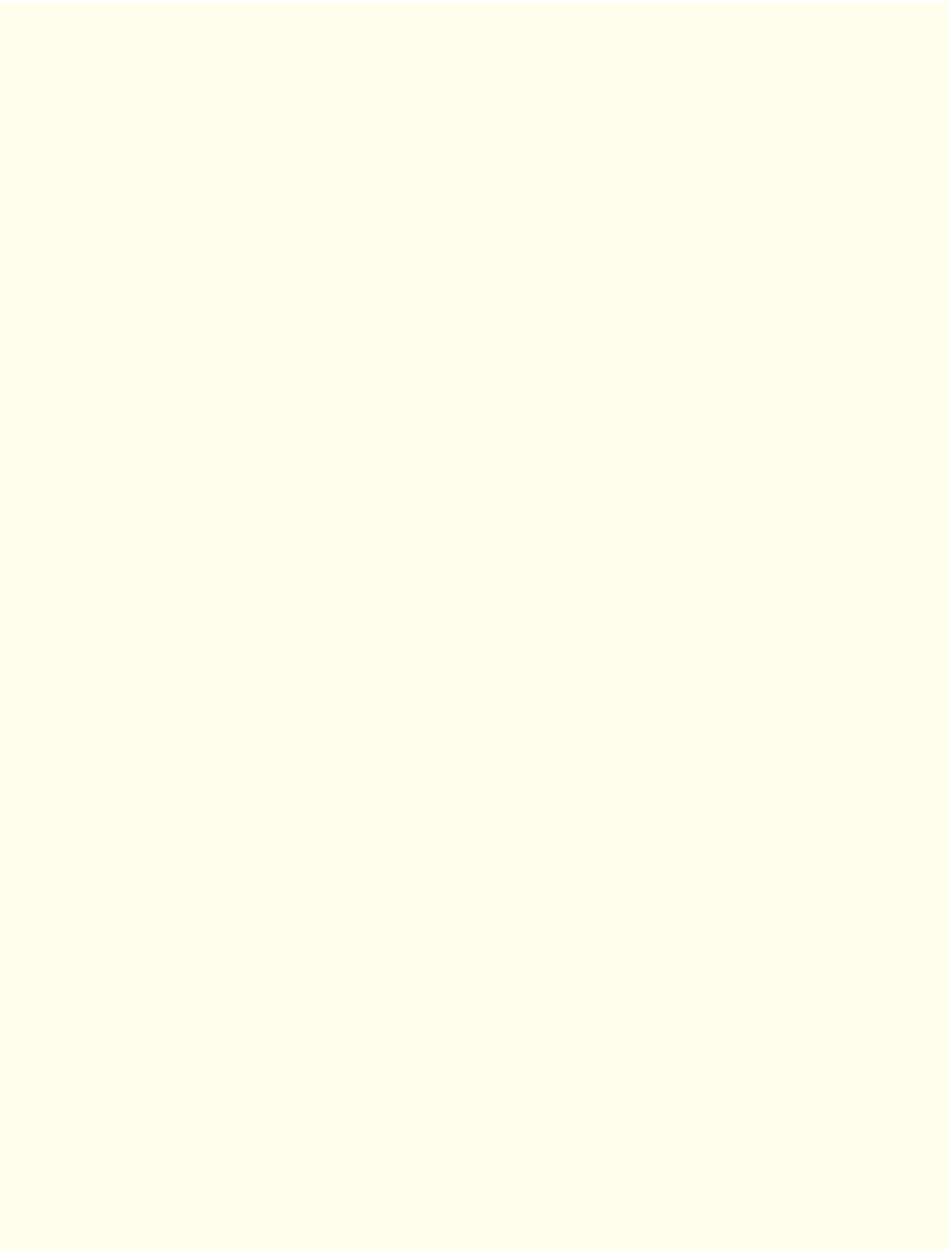