Java Reference
In-Depth Information
performance and are unnecessary for the program's user. To do so, use the
java
com-
mand's
-ea
command-line option, as in
java -ea AssertTest
Software Engineering Observation 11.13
Users shouldn't encounter
AssertionError
s—these should be used only during program
development. For this reason, you shouldn't catch
AssertionError
s. Instread, allow the
program to terminate, so you can see the error message, then locate and fix the source of the
problem. You should not use
assert
to indicate runtime problems in production code (as we
did in Fig. 11.8 for demonstration purposes)—use the exception mechanism for this purpose.
Deallocation
Typically
resource-release code
should be placed in a
finally
block to ensure that a resource
is released, regardless of whether there were exceptions when the resource was used in the
corresponding
try
block. An alternative notation—the
try
-with-resources
statement (in-
troduced in Java SE 7)—simplifies writing code in which you obtain one or more resourc-
es, use them in a
try
block and release them in a corresponding
finally
block. For
example, a file-processing application could process a file with a
try
-with-resources state-
ment to ensure that the file is closed properly when it's no longer needed—we demonstrate
this in Chapter 15. Each resource must be an object of a class that implements the
Auto-
Closeable
interface, and thus provides a
close
method. The general form of a
try
-with-
resources statement is
try
(
ClassName
theObject =
new
ClassName
())
{
// use theObject here
}
catch
(Exception e)
{
// catch exceptions that occur while using the resource
}
where
ClassName
is a class that implements the
AutoCloseable
interface. This code creates
an object of type
ClassName
and uses it in the
try
block, then calls its
close
method to
release any resources used by the object. The
try
-with-resources statement
implicitly
calls
the
theObject
's
close
method
at the end of the
try
block
. You can allocate multiple re-
sources in the parentheses following
try
by separating them with a semicolon (
;
). You'll
see examples of the
try
-with-resources statement in Chapters 15 and 24.
In this chapter, you learned how to use exception handling to deal with errors. You learned
that exception handling enables you to remove error-handling code from the “main line”
of the program's execution. We showed how to use
try
blocks to enclose code that may
throw an exception, and how to use
catch
blocks to deal with exceptions that may arise.
You learned about the termination model of exception handling, which dictates that
after an exception is handled, program control does not return to the throw point. We dis-

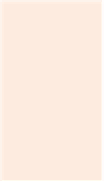



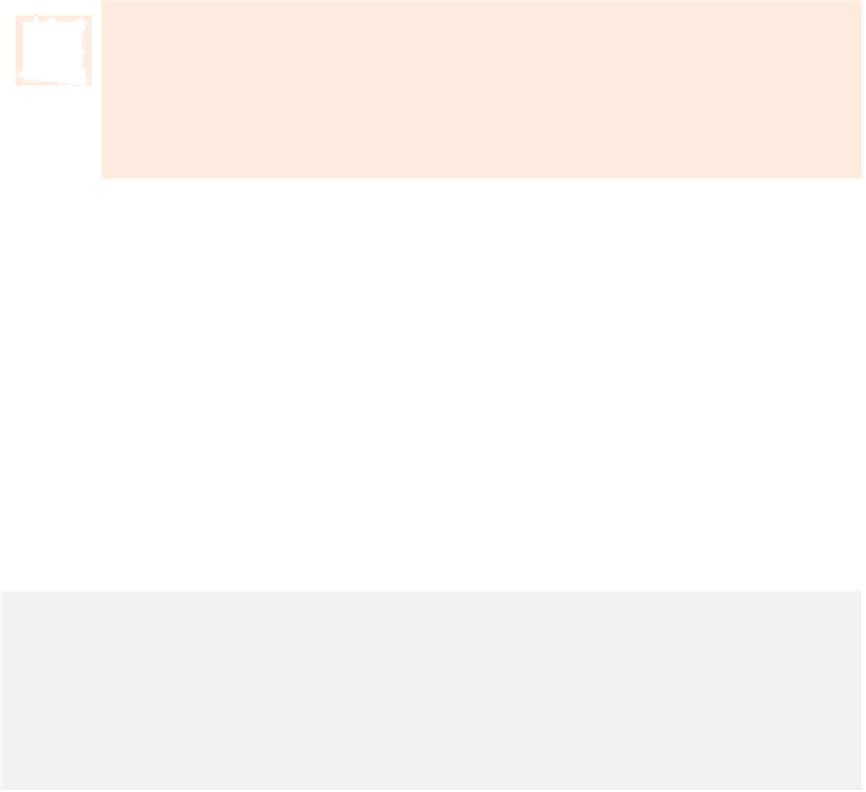