Java Reference
In-Depth Information
11
CPPHTP(
"C++ How to Program"
,
"2014"
),
VBHTP(
"Visual Basic How to Program"
,
"2014"
),
CSHARPHTP(
"Visual C# How to Program"
,
"2014"
);
12
13
14
15
// instance fields
16
private final
String title;
// book title
17
private final
String copyrightYear;
// copyright year
18
19
// enum constructor
20
Book(String title, String copyrightYear)
21
{
22
this
.title = title;
23
this
.copyrightYear = copyrightYear;
24
}
25
26
// accessor for field title
27
public
String getTitle()
28
{
29
return
title;
30
}
31
32
// accessor for field copyrightYear
33
public
String getCopyrightYear()
34
{
35
return
copyrightYear;
36
}
37
}
// end enum Book
Fig. 8.10
|
Declaring an
enum
type with a constructor and explicit instance fields and accessors
for these fields. (Part 2 of 2.)
Using
enum
type
Book
Figure 8.11 tests the
enum
type
Book
and illustrates how to iterate through a range of
enum
constants. For every
enum
, the compiler generates the
static
method
values
(called in
line 12) that returns an array of the
enum
's constants in the order they were declared. Lines
12-14 use the enhanced
for
statement to display all the constants declared in the
enum
Book
. Line 14 invokes the
enum
Book
's
getTitle
and
getCopyrightYear
methods to get
the title and copyright year associated with the constant. When an
enum
constant is con-
verted to a
String
(e.g.,
book
in line 13), the constant's identifier is used as the
String
representation (e.g.,
JHTP
for the first
enum
constant).
1
// Fig. 8.11: EnumTest.java
2
// Testing enum type Book.
3
import
java.util.EnumSet;
4
5
public class
EnumTest
6
{
7
public static void
main(String[] args)
8
{
Fig. 8.11
|
Testing
enum
type
Book
. (Part 1 of 2.)
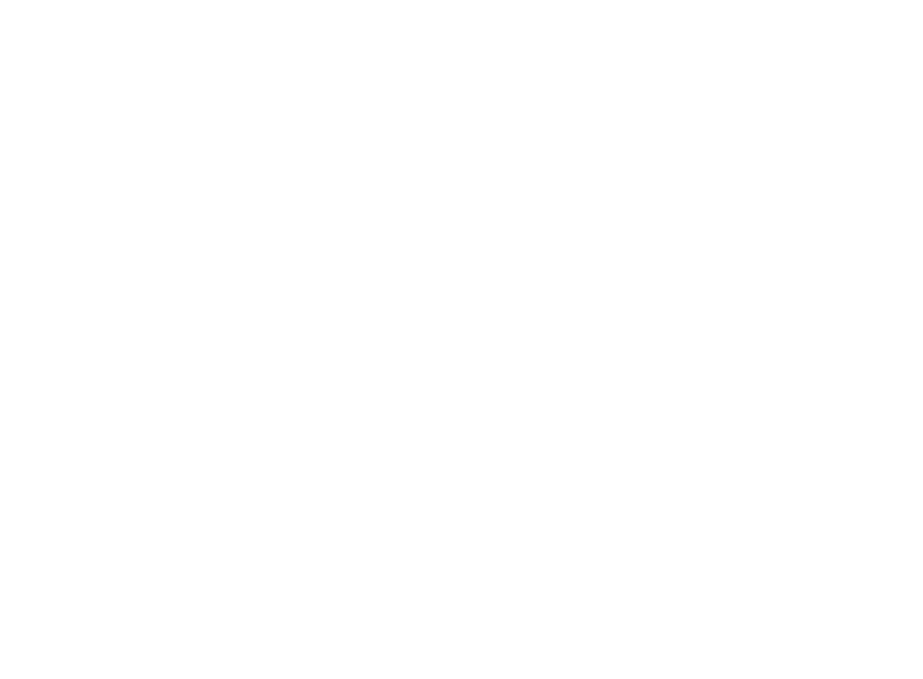
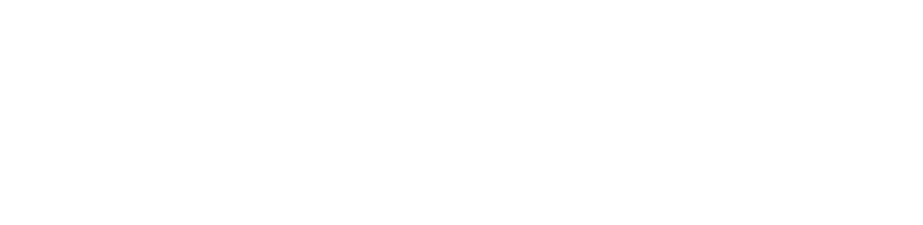






