Java Reference
In-Depth Information
where
parameter
has a type and an identifier (e.g.,
int number
), and
arrayName
is the array
through which to iterate.
• The enhanced
for
statement cannot be used to modify elements in an array. If a program needs
to modify elements, use the traditional counter-controlled
for
statement.
Section 7.8 Passing Arrays to Methods
• When an argument is passed by value, a copy of the argument's value is made and passed to the
called method. The called method works exclusively with the copy.
Section 7.9 Pass-By-Value vs. Pass-By-Reference
• When an argument is passed by reference (p. 265), the called method can access the argument's
value in the caller directly and possibly modify it.
• All arguments in Java are passed by value. A method call can pass two types of values to a meth-
od—copies of primitive values and copies of references to objects. Although an object's reference
is passed by value (p. 265), a method can still interact with the referenced object by calling its
public
methods using the copy of the object's reference.
• To pass an object reference to a method, simply specify in the method call the name of the vari-
able that refers to the object.
• When you pass an array or an individual array element of a reference type to a method, the called
method receives a copy of the array or element's reference. When you pass an individual element
of a primitive type, the called method receives a copy of the element's value.
• To pass an individual array element to a method, use the indexed name of the array.
Section 7.11 Multidimensional Arrays
• Multidimensional arrays with two dimensions are often used to represent tables of values con-
sisting of information arranged in rows and columns.
• A two-dimensional array (p. 272) with
m
rows and
n
columns is called an
m
-by-
n
array. Such an
array can be initialized with an array initializer of the form
arrayType
[][]
arrayName
= {{
row1
initializer
}, {
row2
initializer
},
…
};
• Multidimensional arrays are maintained as arrays of separate one-dimensional arrays. As a result,
the lengths of the rows in a two-dimensional array are not required to be the same.
• A multidimensional array with the same number of columns in every row can be created with an
array-creation expression of the form
arrayType
[][]
arrayName
=
new
arrayType
[
numRows
][
numColumns
];
Section 7.13 Variable-Length Argument Lists
• An argument type followed by an ellipsis (
...
; p. 281) in a method's parameter list indicates that
the method receives a variable number of arguments of that particular type. The ellipsis can occur
only once in a method's parameter list. It must be at the end of the parameter list.
• A variable-length argument list (p. 281) is treated as an array within the method body. The num-
ber of arguments in the array can be obtained using the array's
length
field.
Section 7.14 Using Command-Line Arguments
• Passing arguments to
main
(p. 283) from the command line is achieved by including a parameter
of type
String[]
in the parameter list of
main
. By convention,
main
's parameter is named
args
.
• Java passes command-line arguments that appear after the class name in the
java
command to
the application's
main
method as
String
s in the array
args
.
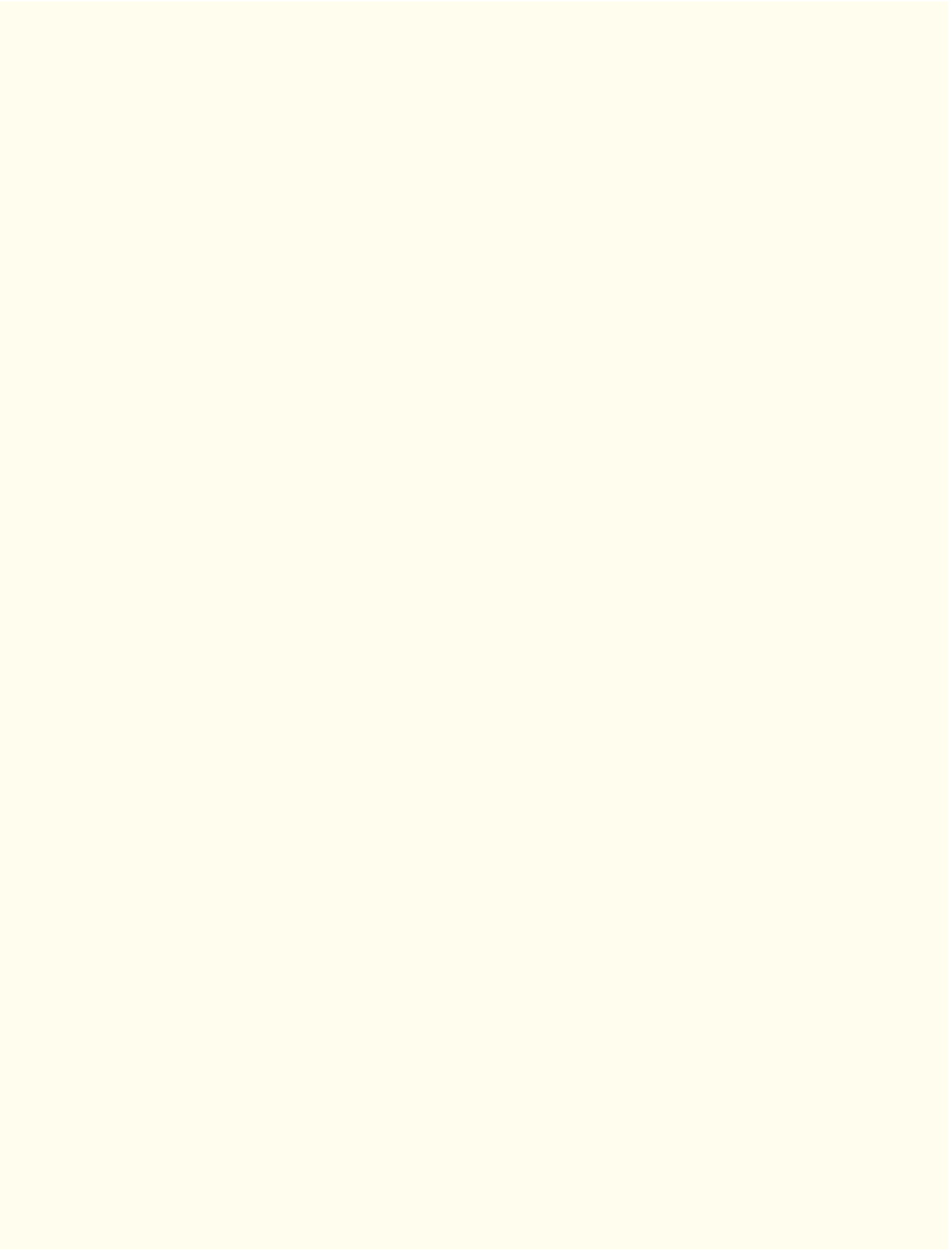