Java Reference
In-Depth Information
ArrayList<T>
(package
java.util
) provides a convenient solution to this problem—it
can
dynamically
change its size to accommodate more elements. The
T
(by convention) is
a
placeholder
—when declaring a new
ArrayList
, replace it with the type of elements that
you want the
ArrayList
to hold. For example,
ArrayList<String> list;
declares
list
as an
ArrayList
collection that can store only
String
s. Classes with this
kind of placeholder that can be used with any type are called
generic classes
.
Only
nonprimitive types can be used to declare variables and create objects of generic classes
. How-
ever, Java provides a mechanism—known as
boxing
—that allows primitive values to be
wrapped as objects for use with generic classes. So, for example,
ArrayList<Integer> integers;
declares
integers
as an
ArrayList
that can store only
Integer
s. When you place an
int
value into an
ArrayList<Integer>
, the
int
value is
boxed
(wrapped) as an
Integer
object,
and when you get an
Integer
object from an
ArrayList<Integer>
, then assign the object
to an
int
variable, the
int
value inside the object is
unboxed
(unwrapped).
Additional generic collection classes and generics are discussed in Chapters 16 and 20,
respectively. Figure 7.23 shows some common methods of class
ArrayList<T>
.
Method
Description
Adds an element to the
end
of the
ArrayList
.
add
Removes all the elements from the
ArrayList
.
clear
Returns
true
if the
ArrayList
contains the specified element; otherwise,
returns
false
.
contains
Returns the element at the specified index.
get
Returns the index of the first occurrence of the specified element in the
ArrayList
.
indexOf
Overloaded. Removes the first occurrence of the specified value or the ele-
ment at the specified index.
remove
Returns the number of elements stored in the
ArrayList
.
size
Trims the capacity of the
ArrayList
to the current number of elements.
trimToSize
Fig. 7.23
|
Some methods and properties of class
ArrayList<T>
.
Demonstrating an
ArrayList<String>
Figure 7.24 demonstrates some common
ArrayList
capabilities. Line 10 creates a new
empty
ArrayList
of
String
s with a default initial capacity of 10 elements. The capacity
indicates how many items the
ArrayList
can hold
without growing
.
ArrayList
is imple-
mented using a conventional array behind the scenes. When the
ArrayList
grows, it must
create a larger internal array and
copy
each element to the new array. This is a time-con-
suming operation. It would be inefficient for the
ArrayList
to grow each time an element
is added. Instead, it grows only when an element is added
and
the number of elements is
equal to the capacity—i.e., there's no space for the new element.
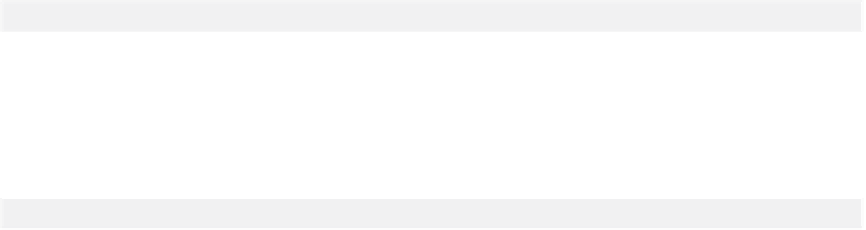
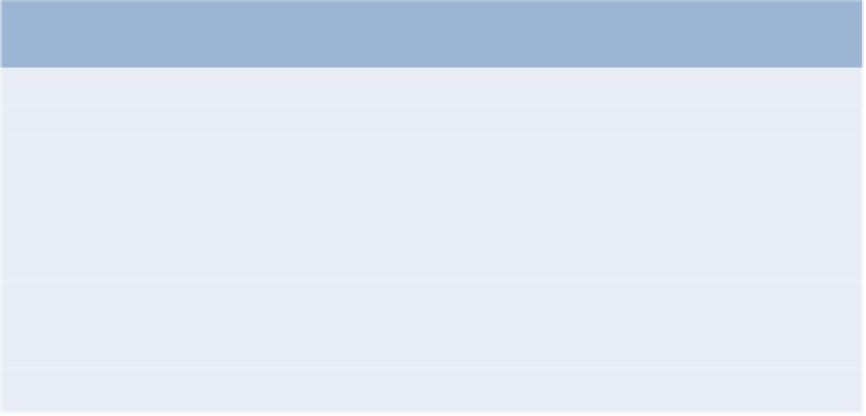