Java Reference
In-Depth Information
It's possible to pass arguments from the command line to an application via method
main
's
String[]
parameter, which receives an array of
String
s. By convention, this parameter is
named
args
. When an application is executed using the
java
command, Java passes the
command-line arguments
that appear after the class name in the
java
command to the
application's
main
method as
String
s in the array
args
. The number of command-line ar-
guments is obtained by accessing the array's
length
attribute. Common uses of command-
line arguments include passing options and filenames to applications.
Our next example uses command-line arguments to determine the size of an array, the
value of its first element and the increment used to calculate the values of the array's
remaining elements. The command
java InitArray 5 0 4
passes three arguments,
5
,
0
and
4
, to the application
InitArray
. Command-line argu-
ments are separated by white space,
not
commas. When this command executes,
InitAr-
ray
's
main
method receives the three-element array
args
(i.e.,
args.length
is
3
) in which
args[0]
contains the
String
"5"
,
args[1]
contains the
String
"0"
and
args[2]
contains
the
String
"4"
. The program determines how to use these arguments—in Fig. 7.21 we
convert the three command-line arguments to
int
values and use them to initialize an ar-
ray. When the program executes, if
args.length
is not
3
, the program prints an error mes-
sage and terminates (lines 9-12). Otherwise, lines 14-32 initialize and display the array
based on the values of the command-line arguments.
Line 16 gets
args[0]
—a
String
that specifies the array size—and converts it to an
int
value that the program uses to create the array in line 17. The
static
method
parseInt
of class
Integer
converts its
String
argument to an
int
.
Lines 20-21 convert the
args[1]
and
args[2]
command-line arguments to
int
values and store them in
initialValue
and
increment
, respectively. Lines 24-25 calcu-
late the value for each array element.
1
// Fig. 7.21: InitArray.java
2
// Initializing an array using command-line arguments.
3
4
public class
InitArray
5
{
6
public static void
main(
String[] args
)
7
{
8
// check number of command-line arguments
9
if
(
args.length !=
3
)
10
System.out.printf(
11
"Error: Please re-enter the entire command, including%n"
+
12
"an array size, initial value and increment.%n"
);
13
else
14
{
15
// get array size from first command-line argument
16
int
arrayLength = Integer.parseInt(args[
0
]);
17
int
[] array =
new
int
[arrayLength];
Fig. 7.21
|
Initializing an array using command-line arguments. (Part 1 of 2.)

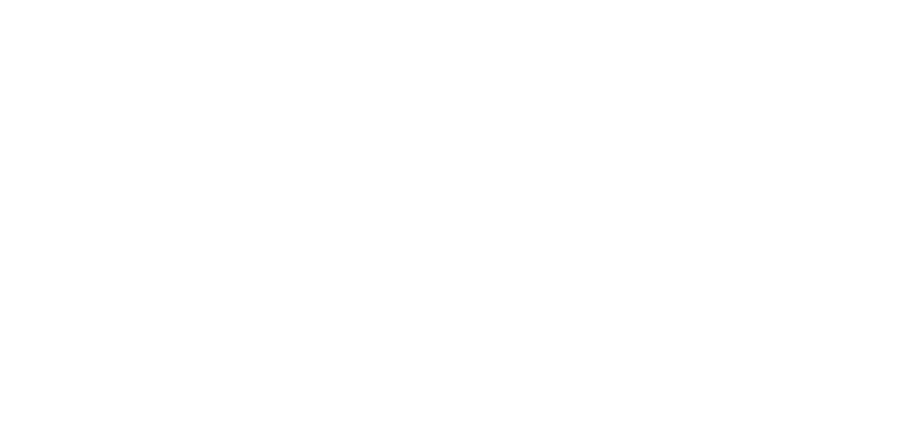










