Java Reference
In-Depth Information
Lines 38-39 in method
processGrades
call methods
getMinimum
and
getMaximum
to
determine the lowest and highest grades of any student on the exam, respectively. Each of
these methods uses an enhanced
for
statement to loop through array
grades
. Lines 51-
56 in method
getMinimum
loop through the array. Lines 54-55 compare each grade to
lowGrade
; if a grade is less than
lowGrade
,
lowGrade
is set to that grade. When line 58 exe-
cutes,
lowGrade
contains the lowest grade in the array. Method
getMaximum
(lines 62-75)
works similarly to method
getMinimum
.
Finally, line 42 in method
processGrades
calls
outputBarChart
to print a grade distri-
bution chart using a technique similar to that in Fig. 7.6. In that example, we manually cal-
culated the number of grades in each category (i.e., 0-9, 10-19, …, 90-99 and 100) by
simply looking at a set of grades. Here, lines 99-100 use a technique similar to that in
Figs. 7.7-7.8 to calculate the frequency of grades in each category. Line 96 declares and cre-
ates array
frequency
of 11
int
s to store the frequency of grades in each category. For each
grade
in array
grades
, lines 99-100 increment the appropriate
frequency
array element. To
determine which one to increment, line 100 divides the current
grade
by 10 using
integer
division
—e.g., if
grade
is
85
, line 100 increments
frequency[8]
to update the count of
grades in the range 80-89. Lines 103-117 print the bar chart (as shown in Fig. 7.15) based
on the values in array
frequency
. Like lines 23-24 of Fig. 7.6, lines 113-116 of Fig. 7.14
use a value in array
frequency
to determine the number of asterisks to display in each bar.
Class
GradeBookTest
That Demonstrates Class
GradeBook
The application of Fig. 7.15 creates an object of class
GradeBook
(Fig. 7.14) using the
int
array
gradesArray
(declared and initialized in line 10). Lines 12-13 pass a course name and
gradesArray
to the
GradeBook
constructor. Lines 14-15 display a welcome message that in-
cludes the course name stored in the
GradeBook
object. Line 16 invokes the
GradeBook
ob-
ject's
processGrades
method. The output summarizes the 10 grades in
myGradeBook
.
Software Engineering Observation 7.2
A test harness (or test application) is responsible for creating an object of the class being
tested and providing it with data. This data could come from any of several sources. Test
data can be placed directly into an array with an array initializer, it can come from the
user at the keyboard, from a file (as you'll see in Chapter 15), from a database (as you'll
see in Chapter 24) or from a network (as you'll see in online Chapter 28). After passing
this data to the class's constructor to instantiate the object, the test harness should call upon
the object to test its methods and manipulate its data. Gathering data in the test harness
like this allows the class to be more reusable, able to manipulate data from several sources.
1
// Fig. 7.15: GradeBookTest.java
2
// GradeBookTest creates a GradeBook object using an array of grades,
3
// then invokes method processGrades to analyze them.
4
public
class
GradeBookTest
5
{
6
// main method begins program execution
7
public
static
void
main(String[] args)
8
{
Fig. 7.15
|
GradeBookTest
creates a
GradeBook
object using an array of grades, then invokes
method
processGrades
to analyze them. (Part 1 of 2.)


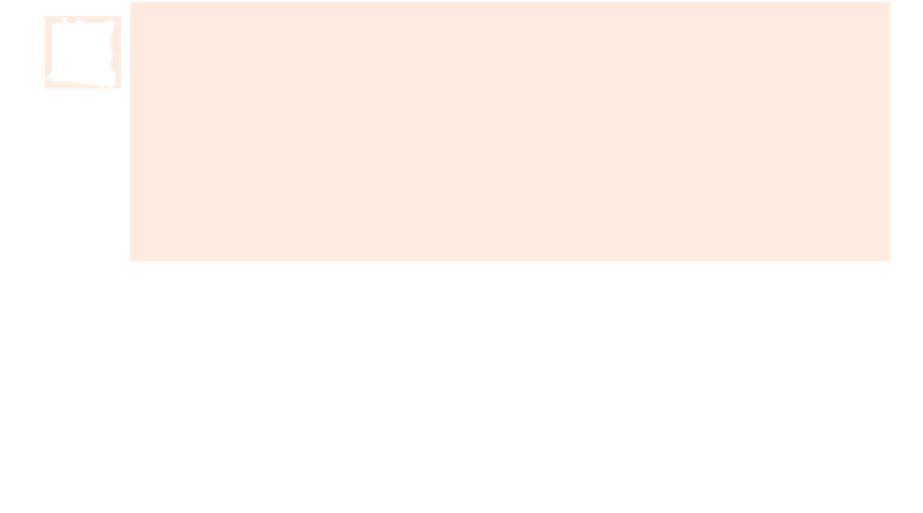

