Java Reference
In-Depth Information
Figure 7.13 demonstrates the difference between passing an entire array and passing
a primitive-type array element to a method. Notice that
main
invokes
static
methods
modifyArray
(line 19) and
modifyElement
(line 30) directly. Recall from Section 6.4 that
a
static
method of a class can invoke other
static
methods of the same class directly.
The enhanced
for
statement at lines 16-17 outputs the elements of
array
. Line 19
invokes method
modifyArray
, passing
array
as an argument. The method (lines 36-40)
receives a copy of
array
's reference and uses it to multiply each of
array
's elements by 2. To
prove that
array
's elements were modified, lines 23-24 output
array
's elements again. As
the output shows, method
modifyArray
doubled the value of each element. We could
not
use the enhanced
for
statement in lines 38-39 because we're modifying the array's elements.
1
// Fig. 7.13: PassArray.java
2
// Passing arrays and individual array elements to methods.
3
4
public class
PassArray
5
{
6
// main creates array and calls modifyArray and modifyElement
7
public static void
main(String[] args)
8
{
9
int
[] array = {
1
,
2
,
3
,
4
,
5
};
10
11
System.out.printf(
12
"Effects of passing reference to entire array:%n"
+
13
"The values of the original array are:%n"
);
14
15
// output original array elements
16
for
(
int
value : array)
17
System.out.printf(
" %d"
, value);
18
19
modifyArray(array);
// pass array reference
20
System.out.printf(
"%n%nThe values of the modified array are:%n"
);
21
22
// output modified array elements
23
for
(
int
value : array)
24
System.out.printf(
" %d"
, value);
25
26
System.out.printf(
27
"%n%nEffects of passing array element value:%n"
+
28
"array[3] before modifyElement: %d%n"
, array[
3
]);
29
30
modifyElement(array[
3
]);
// attempt to modify array[3]
31
System.out.printf(
32
"array[3] after modifyElement: %d%n"
, array[
3
]);
33
}
34
35
// multiply each element of an array by 2
public static void
modifyArray(
int
[] array2)
{
for
(
int
counter =
0
; counter < array2.length; counter++)
array2[counter] *=
2
;
}
36
37
38
39
40
Fig. 7.13
|
Passing arrays and individual array elements to methods. (Part 1 of 2.)
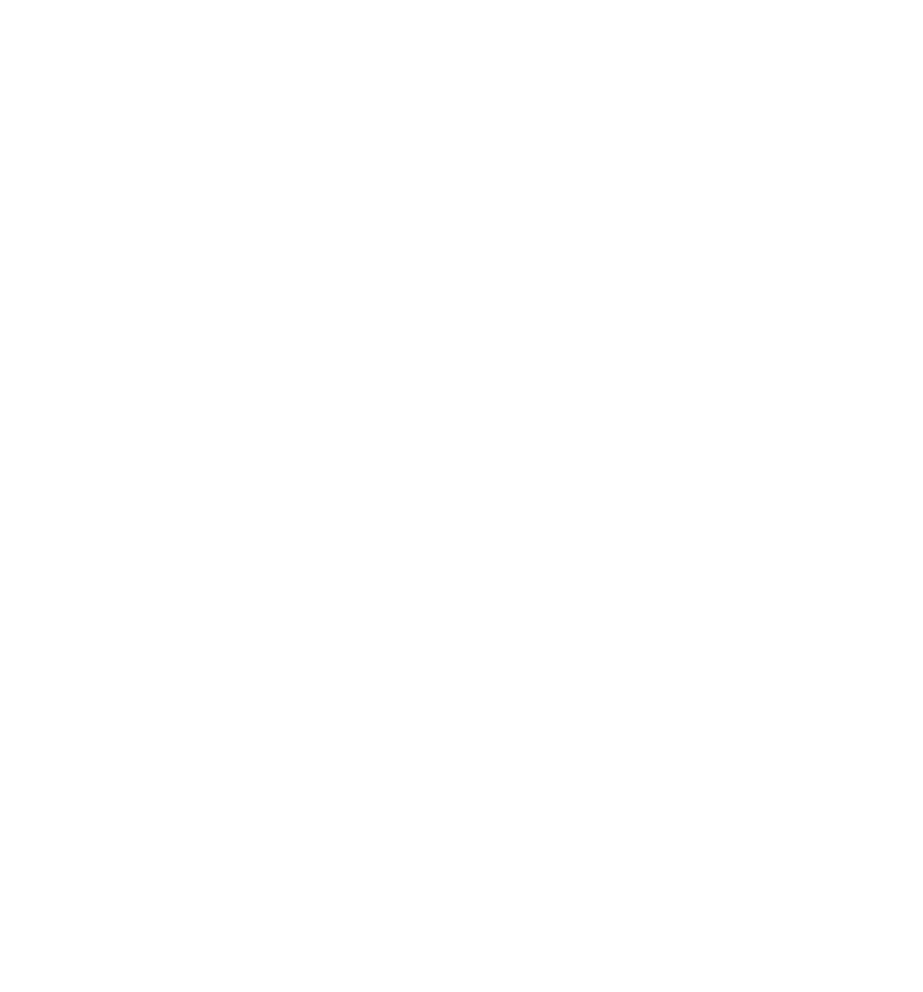

























