Java Reference
In-Depth Information
c)
Error: The semicolon after the right parenthesis of the parameter list is incorrect, and
the parameter
a
should not be redeclared in the method.
Correction: Delete the semicolon after the right parenthesis of the parameter list, and
delete the declaration
float a;
.
d)
Error: The method returns a value when it's not supposed to.
Correction: Change the return type from
void
to
int
.
6.6
The following solution calculates the volume of a sphere, using the radius entered by the user:
1
// Exercise 6.6: Sphere.java
2
// Calculate the volume of a sphere.
3
import
java.util.Scanner;
4
5
public class
Sphere
6
{
7
// obtain radius from user and display volume of sphere
8
public static void
main(String[] args)
9
{
10
Scanner input =
new
Scanner(System.in);
11
12
System.out.print(
"Enter radius of sphere: "
);
13
double
radius = input.nextDouble();
14
15
System.out.printf(
"Volume is %f%n"
, sphereVolume(radius));
16
}
// end method determineSphereVolume
17
18
// calculate and return sphere volume
19
public static double
sphereVolume(
double
radius)
20
{
21
double
volume = (
4.0
/
3.0
) *
Math.PI
* Math.pow(radius,
3
);
22
return
volume;
23
}
// end method sphereVolume
24
}
// end class Sphere
Enter radius of sphere:
4
Volume is 268.082573
Exercises
6.7
What is the value of
x
after each of the following statements is executed?
a)
x = Math.abs(
7.5
);
b)
x = Math.floor(
7.5
)
;
c)
x = Math.abs(
0.0
);
d)
x = Math.ceil(
0.0
);
e)
x = Math.abs(
-6.4
)
;
f)
x = Math.ceil(
-6.4
);
g)
x = Math.ceil(-Math.abs(
-8
+ Math.floor(
-5.5
)));
6.8
(Parking Charges)
A parking garage charges a $2.00 minimum fee to park for up to three
hours. The garage charges an additional $0.50 per hour for each hour
or part thereof
in excess of three
hours. The maximum charge for any given 24-hour period is $10.00. Assume that no car parks for
longer than 24 hours at a time. Write an application that calculates and displays the parking charges
for each customer who parked in the garage yesterday. You should enter the hours parked for each
customer. The program should display the charge for the current customer and should calculate and
display the running total of yesterday's receipts. It should use the method
calculateCharges
to de-
termine the charge for each customer.
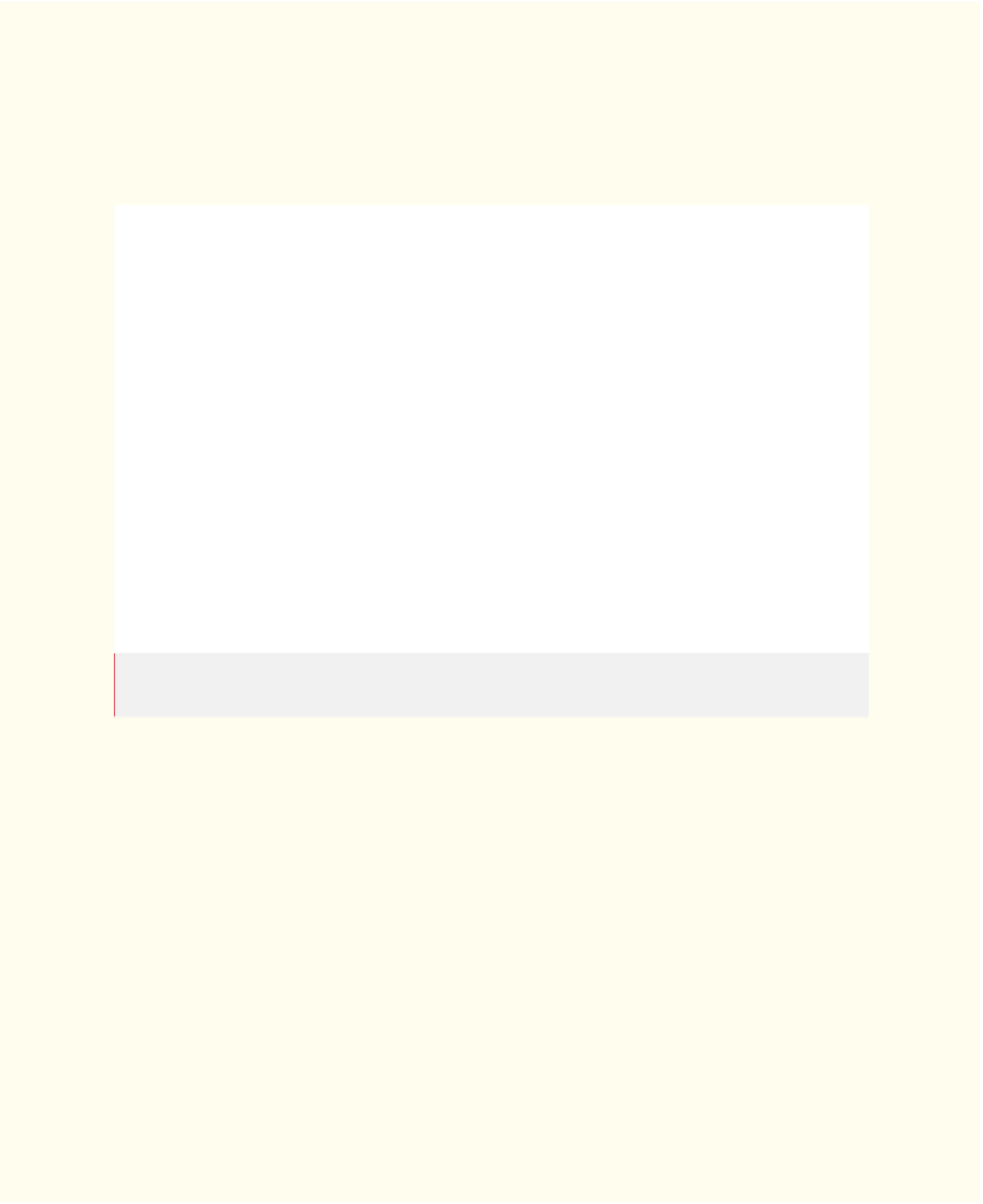



