Java Reference
In-Depth Information
6
// test overloaded square methods
7
public static void
main(String[] args)
8
{
9
System.out.printf(
"Square of integer 7 is %d%n"
,
square(
7
)
square(
7.5
)
);
10
System.out.printf(
"Square of double 7.5 is %f%n"
,
);
11
}
12
13
// square method with int argument
14
public static int
square(
int
intValue)
15
{
16
System.out.printf(
"%nCalled square with int argument: %d%n"
,
17
intValue);
18
return
intValue * intValue;
19
}
20
21
// square method with double argument
22
public static double
square(
double
doubleValue)
23
{
24
System.out.printf(
"%nCalled square with double argument: %f%n"
,
25
doubleValue);
26
return
doubleValue * doubleValue;
27
}
28
}
// end class MethodOverload
Called square with int argument: 7
Square of integer 7 is 49
Called square with double argument: 7.500000
Square of double 7.5 is 56.250000
Fig. 6.10
|
Overloaded method declarations. (Part 2 of 2.)
Line 9 invokes method
square
with the argument
7
. Literal integer values are treated
as type
int
, so the method call in line 9 invokes the version of
square
at lines 14-19 that
specifies an
int
parameter. Similarly, line 10 invokes method
square
with the argument
7.5
. Literal floating-point values are treated as type
double
, so the method call in line 10
invokes the version of
square
at lines 22-27 that specifies a
double
parameter. Each
method first outputs a line of text to prove that the proper method was called in each case.
The values in lines 10 and 24 are displayed with the format specifier
%f
. We did not specify
a precision in either case. By default, floating-point values are displayed with six digits of
precision if the precision is
not
specified in the format specifier.
Distinguishing Between Overloaded Methods
The compiler distinguishes overloaded methods by their
signatures
—a combination of the
method's
name
and the
number
,
types
and
order
of its parameters, but
not
its return type. If
the compiler looked only at method names during compilation, the code in Fig. 6.10 would
be ambiguous—the compiler would not know how to distinguish between the two
square
methods (lines 14-19 and 22-27). Internally, the compiler uses longer method names that
include the original method name, the types of each parameter and the exact order of the
parameters to determine whether the methods in a class are
unique
in that class.
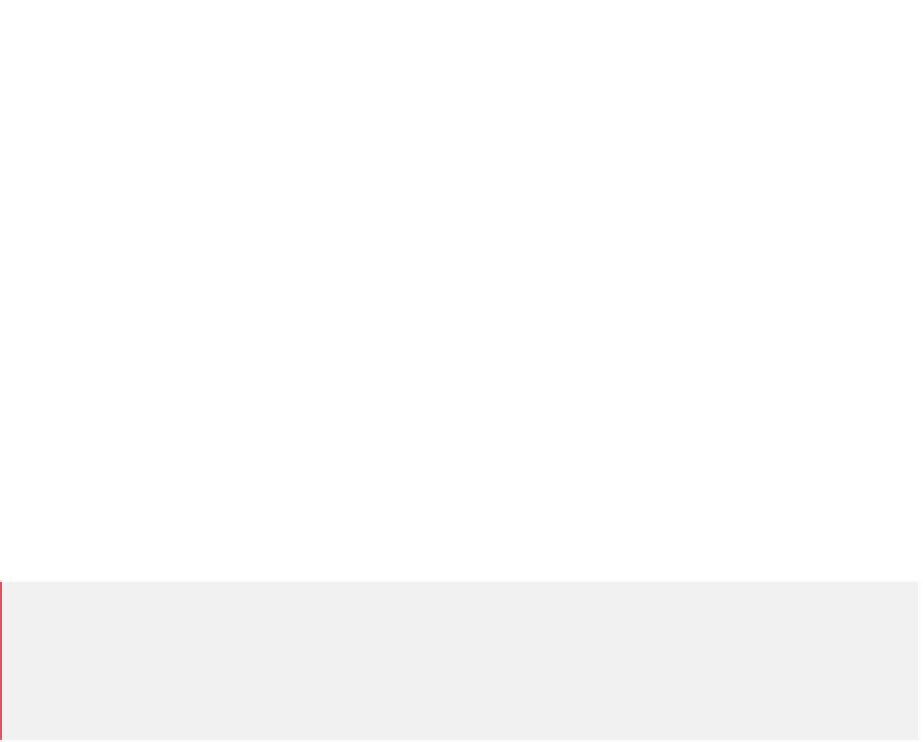




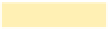
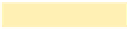

