Java Reference
In-Depth Information
Section 5.2 presented the essentials of counter-controlled repetition. The
while
statement
can be used to implement any counter-controlled loop. Java also provides the
for
repeti-
tion statement
, which specifies the counter-controlled-repetition details in a single line of
code. Figure 5.2 reimplements the application of Fig. 5.1 using
for
.
1
// Fig. 5.2: ForCounter.java
2
// Counter-controlled repetition with the for repetition statement.
3
4
public class
ForCounter
5
{
6
public static void
main(String[] args)
7
{
8
// for statement header includes initialization,
// loop-continuation condition and increment
for
(
int
counter =
1
; counter <=
10
; counter++)
System.out.printf(
"%d "
, counter);
9
10
11
12
13
System.out.println();
14
}
15
}
// end class ForCounter
1 2 3 4 5 6 7 8 9 10
Fig. 5.2
|
Counter-controlled repetition with the
for
repetition statement.
When the
for
statement (lines 10-11) begins executing, the control variable
counter
is
declared
and
initialized
to
1
. (Recall from Section 5.2 that the first two elements of
counter-controlled repetition are the
control variable
and its
initial value
.) Next, the pro-
gram checks the
loop-continuation condition
,
counter <= 10
, which is between the two
required semicolons. Because the initial value of
counter
is
1
, the condition initially is
true. Therefore,
the
body
statement (line 11)
displays control variable
counter
's value,
namely
1
. After executing the loop's body, the program increments
counter
in the expres-
sion
counter++
, which appears to the right of the second semicolon. Then the loop-con-
tinuation test is performed again to determine whether the program should continue with
the next iteration of the loop. At this point, the control variable's value is
2
, so the condi-
tion is still true (the
final value
is not exceeded)—thus, the program performs the body
statement again (i.e., the next iteration of the loop). This process continues until the num-
bers 1 through 10 have been displayed and the
counter
's value becomes
11
, causing the
loop-continuation test to fail and repetition to terminate (after 10 repetitions of the loop
body). Then the program performs the first statement after the
for
—in this case, line 13.
Figure 5.2 uses (in line 10) the loop-continuation condition
counter
<=
10
. If you
incorrectly specified
counter
<
10
as the condition, the loop would iterate only nine times.
This is a common
logic error
called an
off-by-one error
.
Common Programming Error 5.2
Using an incorrect relational operator or an incorrect final value of a loop counter in the
loop-continuation condition of a repetition statement can cause an off-by-one error.
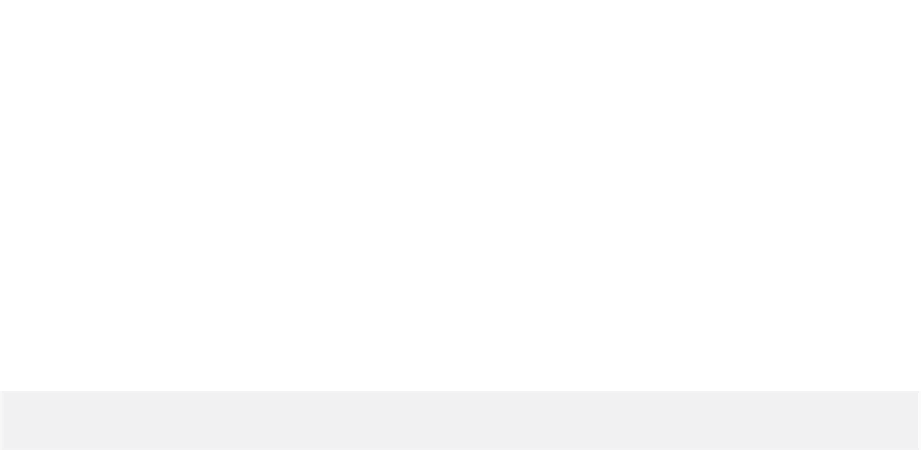









