Java Reference
In-Depth Information
4
Prompt the user to enter the first grade
5
Input the first grade (possibly the sentinel)
6
7
While the user has not yet entered the sentinel
8
Add this grade into the running total
9
Add one to the grade counter
10
Prompt the user to enter the next grade
11
Input the next grade (possibly the sentinel)
12
13
If the counter is not equal to zero
14
Set the average to the total divided by the counter
15
Print the average
16
else
17
Print “No grades were entered”
Fig. 4.9
|
Class-average pseudocode algorithm with sentinel-controlled repetition. (Part 2 of 2.)
In Fig. 4.7 and Fig. 4.9, we included blank lines and indentation in the pseudocode
to make it more readable. The blank lines separate the algorithms into their phases and set
off control statements; the indentation emphasizes the bodies of the control statements.
The pseudocode algorithm in Fig. 4.9 solves the more general class-average problem.
This algorithm was developed after two refinements. Sometimes more are needed.
Software Engineering Observation 4.5
Terminate the top-down, stepwise refinement process when you've specified the pseudocode
algorithm in sufficient detail for you to convert the pseudocode to Java. Normally,
implementing the Java program is then straightforward.
Software Engineering Observation 4.6
Some programmers do not use program development tools like pseudocode. They feel that
their ultimate goal is to solve the problem on a computer and that writing pseudocode
merely delays the production of final outputs. Although this may work for simple and
familiar problems, it can lead to serious errors and delays in large, complex projects.
Implementing Sentinel-Controlled Repetition
In Fig. 4.10, method
main
(lines 7-46) implements the pseudocode algorithm of Fig. 4.9.
Although each grade is an integer, the averaging calculation is likely to produce a number
with a
decimal point
—in other words, a real (floating-point) number. The type
int
cannot
represent such a number, so this class uses type
double
to do so. You'll also see that control
statements may be
stacked
on top of one another (in sequence). The
while
statement (lines
22-30) is followed in sequence by an
if
…
else
statement (lines 34-45). Much of the code
in this program is identical to that in Fig. 4.8, so we concentrate on the new concepts.
1
// Fig. 4.10: ClassAverage.java
2
// Solving the class-average problem using sentinel-controlled repetition.
3
import
java.util.Scanner;
// program uses class Scanner
Fig. 4.10
|
Solving the class-average problem using sentinel-controlled repetition. (Part 1 of 2.)

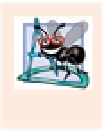



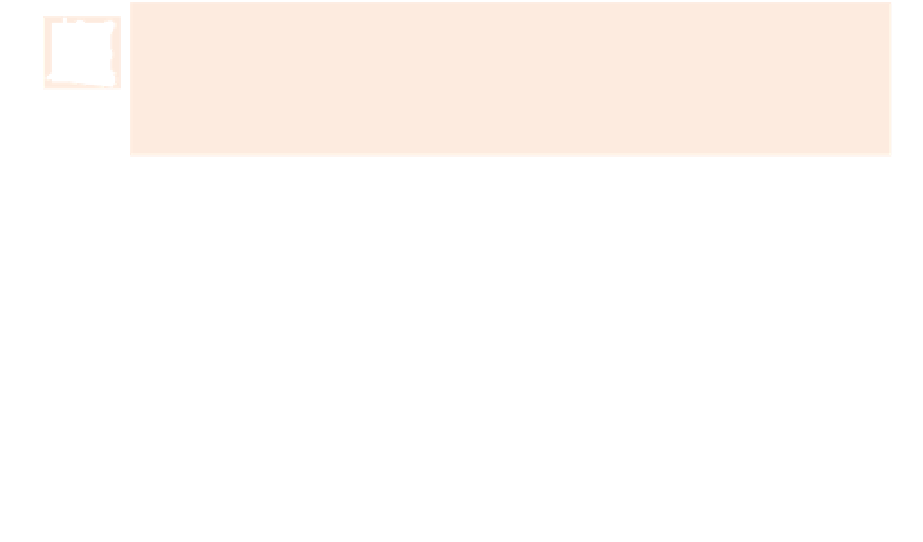



