Java Reference
In-Depth Information
method) form a
block
. A block can be placed anywhere in a method that a single statement
can be placed.
The following example includes a block in the
else
part of an
if
…
else
statement:
if
(grade >=
60
)
System.out.println(
"Passed"
);
else
{
System.out.println(
"Failed"
);
System.out.println(
"You must take this course again.")
;
}
In this case, if
grade
is less than 60, the program executes
both
statements in the body of
the
else
and prints
Failed
You must take this course again.
Note the braces surrounding the two statements in the
else
clause. These braces are im-
portant. Without the braces, the statement
System.out.println(
"You must take this course again."
);
would be outside the body of the
else
part of the
if
…
else
statement and would execute
regardless
of whether the grade was less than 60.
Syntax errors
(e.g., when one brace in a block is left out of the program) are caught by
the compiler. A
logic error
(e.g., when both braces in a block are left out of the program)
has its effect at execution time. A
fatal logic error
causes a program to fail and terminate
prematurely. A
nonfatal logic error
allows a program to continue executing but causes it
to produce incorrect results.
Just as a block can be placed anywhere a single statement can be placed, it's also pos-
sible to have an empty statement. Recall from Section 2.8 that the empty statement is rep-
resented by placing a semicolon (
;
) where a statement would normally be.
Common Programming Error 4.1
Placing a semicolon after the condition in an
if
or
if
…
else
statement leads to a logic
error in single-selection
if
statements and a syntax error in double-selection
if
…
else
statements (when the
if
-part contains an actual body statement).
Conditional Operator (
?:
)
Java provides the
conditional operator
(
?:
)
that can be used in place of an
if
…
else
statement. This can make your code shorter and clearer. The conditional operator is Java's
only
ternary operator
(i.e., an operator that takes
three
operands). Together, the operands
and the
?:
symbol form a
conditional expression.
The first operand (to the left of the
?
)
is a
boolean
expression
(i.e., a
condition
that evaluates to a
boolean
value—
true
or
false
), the second operand (between the
?
and
:
) is the value of the conditional expres-
sion if the
boolean
expression is
true
and the third operand (to the right of the
:
) is the
value of the conditional expression if the
boolean
expression evaluates to
false
. For ex-
ample, the statement
System.out.println(studentGrade
>=
60
?
"Passed"
:
"Failed"
);
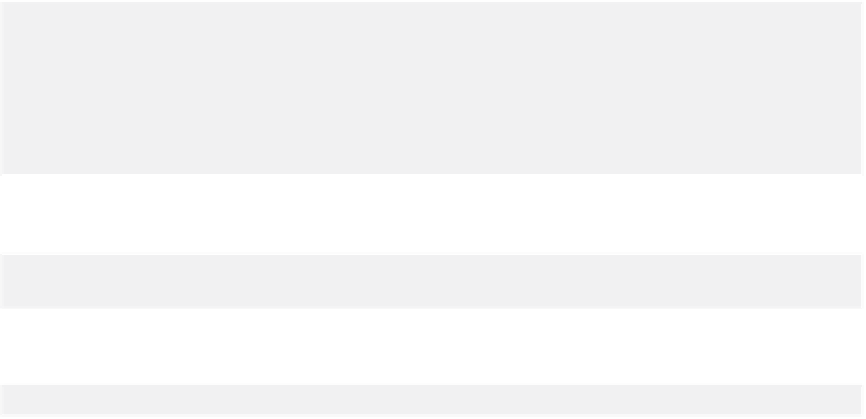

