Java Reference
In-Depth Information
1
// Fig. 3.12: Dialog1.java
2
// Using JOptionPane to display multiple lines in a dialog box.
3
import
javax.swing.JOptionPane;
4
5
public
class
Dialog1
6
{
7
public
static
void
main(String[] args)
8
{
9
// display a dialog with a message
JOptionPane.showMessageDialog(
null
,
"Welcome to Java"
);
10
11
}
12
}
// end class Dialog1
Fig. 3.12
|
Using
JOptionPane
to display multiple lines in a dialog box.
JOptionPane
Class
static
Method
showMessagDialog
Line 3 indicates that the program uses class
JOptionPane
from package
javax.swing
. This
package contains many classes that help you create
graphical user interfaces
(GUIs)
.
GUI
components
facilitate data entry by a program's user and presentation of outputs to the
user. Line 10 calls
JOptionPane
method
showMessageDialog
to display a dialog box con-
taining a message. The method requires two arguments. The first helps the Java app de-
termine where to position the dialog box. A dialog is typically displayed from a GUI app
with its own window. The first argument refers to that window (known as the
parent win-
dow
) and causes the dialog to appear centered over the app's window. If the first argument
is
null
, the dialog box is displayed at the center of your screen. The second argument is
the
String
to display in the dialog box.
Introducing
static
Methods
JOptionPane
method
showMessageDialog
is a so-called
static
method
. Such methods
often define frequently used tasks. For example, many programs display dialog boxes, and
the code to do this is the same each time. Rather than requiring you to “reinvent the
wheel” and create code to display a dialog, the designers of class
JOptionPane
declared a
static
method that performs this task for you. A
static
method is called by using its class
name followed by a dot (
.
) and the method name, as in
ClassName
.
methodName
(
arguments
)
Notice that you do
not
create an object of class
JOptionPane
to use its
static
method
showMessageDialog
.We discuss
static
methods in more detail in Chapter 6.
Entering Text in a Dialog
Figure 3.13 uses another predefined
JOptionPane
dialog called an
input dialog
that allows
the user to
enter data
into a program. The program asks for the user's name and responds
with a message dialog containing a greeting and the name that the user entered.
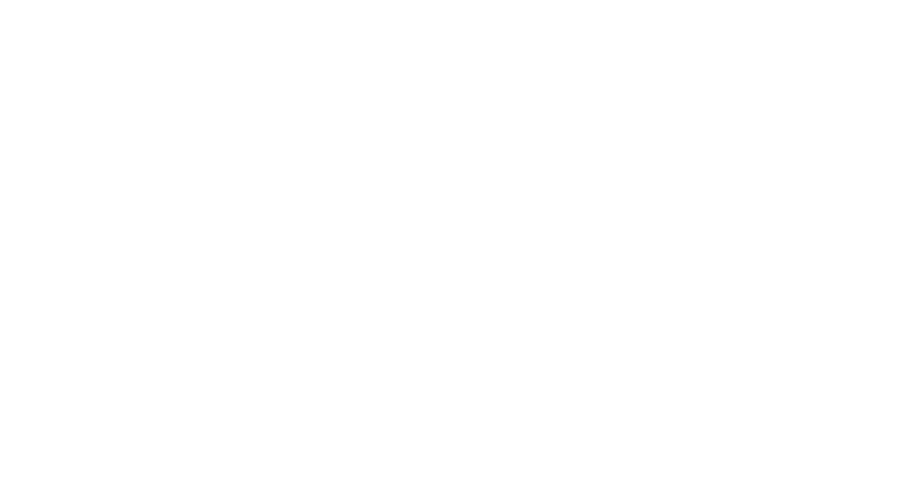
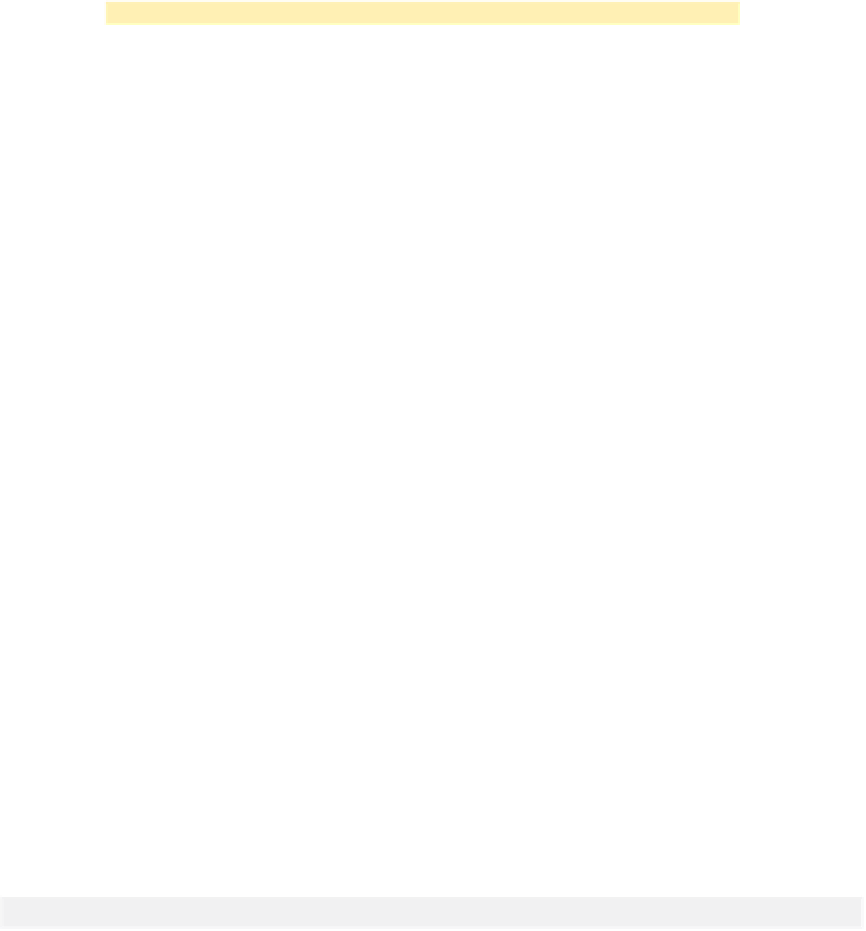

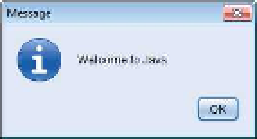





