Java Reference
In-Depth Information
There's No Default Constructor in a Class That Declares a Constructor
If you declare a constructor for a class, the compiler will
not
create a
default constructor
for
that class. In that case, you will not be able to create an
Account
object with the class in-
stance creation expression
new Account()
as we did in Fig. 3.2—unless the custom con-
structor you declare takes
no
parameters.
Software Engineering Observation 3.3
Unless default initialization of your class's instance variables is acceptable, provide a
custom constructor to ensure that your instance variables are properly initialized with
meaningful values when each new object of your class is created.
Adding the Constructor to Class
Account
's UML Class Diagram
The UML class diagram of Fig. 3.7 models class
Account
of Fig. 3.5, which has a construc-
tor with a
String
name
parameter. Like operations, the UML models constructors in the
third
compartment of a class diagram. To distinguish a constructor from the class's oper-
ations, the UML requires that the word “constructor” be enclosed in
guillemets (« and »)
and placed before the constructor's name. It's customary to list constructors
before
other
operations in the third compartment.
Account
- name : String
«constructor» Account(name: String)
+ setName(name: String)
+ getName() : String
Fig. 3.7
|
UML class diagram for
Account
class of Fig. 3.5.
Numbers
We now declare an
Account
class that maintains the
balance
of a bank account in addition
to the name. Most account balances are not integers. So, class
Account
represents the ac-
count balance as a
floating-point number
—a number with a
decimal point
, such as 43.95,
0.0, -129.8873. [In Chapter 8, we'll begin representing monetary amounts precisely with
class
BigDecimal
as you should do when writing industrial-strength monetary applications.]
Java provides two primitive types for storing floating-point numbers in memory—
float
and
double
. Variables of type
float
represent
single-precision floating-point num-
bers
and can hold up to
seven significant digits
. Variables of type
double
represent
double-
precision floating-point numbers
. These require
twice
as much memory as
float
variables
and can hold up to
15 significant digits
—about
double
the precision of
float
variables.
Most programmers represent floating-point numbers with type
double
. In fact, Java
treats all floating-point numbers you type in a program's source code (such as 7.33 and
0.0975) as
double
values by default. Such values in the source code are known as
floating-
point literals
. See Appendix D, Primitive Types, for the precise ranges of values for
float
s
and
double
s.
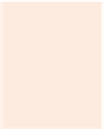






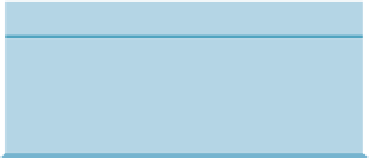