Java Reference
In-Depth Information
Exercises
2.7
Fill in the blanks in each of the following statements:
a)
are used to document a program and improve its readability.
b)
A decision can be made in a Java program with a(n)
.
c)
Calculations are normally performed by
statements.
d)
The arithmetic operators with the same precedence as multiplication are
and
.
e)
When parentheses in an arithmetic expression are nested, the
set of paren-
theses is evaluated first.
f)
A location in the computer's memory that may contain different values at various times
throughout the execution of a program is called a(n)
.
2.8
Write Java statements that accomplish each of the following tasks:
a) Display the message
"Enter an integer: "
, leaving the cursor on the same line.
b) Assign the product of variables
b
and
c
to variable
a
.
c) Use a comment to state that a program performs a sample payroll calculation.
2.9
State whether each of the following is
true
or
false
. If
false
, explain why.
a) Java operators are evaluated from left to right.
b) The following are all valid variable names:
_under_bar_
,
m928134
,
t5
,
j7
,
her_sales$
,
his_$account_total
,
a
,
b$
,
c
,
z
and
z2
.
c) A valid Java arithmetic expression with no parentheses is evaluated from left to right.
d) The following are all invalid variable names:
3g
,
87
,
67h2
,
h22
and
2h
.
2.10
Assuming that
x
=
2
and
y
=
3
, what does each of the following statements display?
a)
System.out.printf(
"x = %d%n"
, x);
b)
System.out.printf(
"Value of %d + %d is %d%n"
, x, x, (x + x));
c)
System.out.printf(
"x ="
);
d)
System.out.printf(
"%d = %d%n"
, (x + y), (y + x));
2.11
Which of the following Java statements contain variables whose values are modified?
a)
p = i + j + k +
7
;
b)
System.out.println(
"variables whose values are modified"
);
c)
System.out.println(
"a = 5"
);
d)
value = input.nextInt();
2.12
Given that
y = ax
3
+ 7, which of the following are correct Java statements for this equation?
a)
y = a * x * x * x +
7
;
b)
y = a * x * x * (x +
7
);
c)
y = (a * x) * x * (x +
7
);
d)
y = (a * x) * x * x +
7
;
e)
y = a * (x * x * x) +
7
;
f)
y = a * x * (x * x +
7
);
2.13
State the order of evaluation of the operators in each of the following Java statements, and
show the value of
x
after each statement is performed:
a)
x =
7
+
3
*
6
/
2
-
1
;
b)
x =
2
%
2
+
2
*
2
-
2
/
2
;
c)
x = (
3
*
9
* (
3
+ (
9
*
3
/ (
3
))));
2.14
Write an application that displays the numbers 1 to 4 on the same line, with each pair of
adjacent numbers separated by one space. Use the following techniques:
a)
Use one
System.out.println
statement.
b)
Use four
System.out.print
statements.
c)
Use one
System.out.printf
statement.
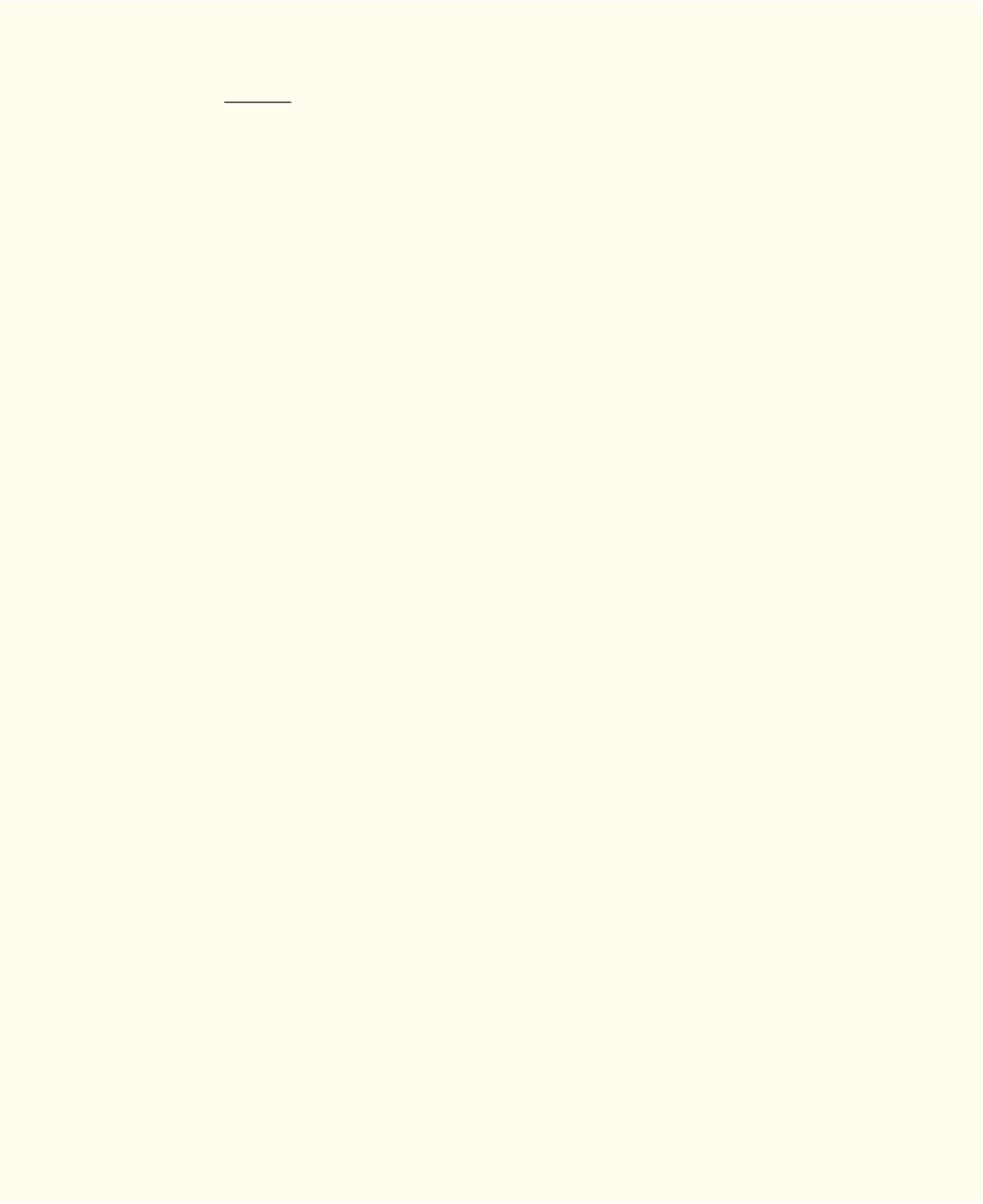




