Java Reference
In-Depth Information
to stop the computation before it completes. Line 91 executes the
PrimeCalculator
to
begin finding primes. If the user clicks the
cancelJButton
, the event handler registered at
lines 107-115 calls
PrimeCalculator
's method
cancel
(line 112), which is inherited from
class
SwingWorker
, and the calculation returns early. The argument
true
to method
cancel
indicates that the thread performing the task should be interrupted in an attempt
to cancel the task.
Date/Time API
In Section 7.15, we used class
Arrays
's
static
method
sort
to sort an array and we in-
troduced
static
method
parallelSort
for sorting large arrays more efficiently on multi-
core systems. Figure 23.28 uses both methods to sort 15,000,000 element arrays of ran-
dom
int
values so that we can demonstrate
parallelSort
's performance improvement of
over
sort
on a multi-core system (we ran this on a dual-core system).
1
// SortComparison.java
2
// Comparing performance of Arrays methods sort and parallelSort.
3
import
java.time.Duration;
4
import
java.time.Instant;
5
import
java.text.NumberFormat;
6
import
java.util.Arrays;
7
import
java.security.SecureRandom;
8
9
public class
SortComparison
10
{
11
public static void
main(String[] args)
12
{
13
SecureRandom random =
new
SecureRandom();
14
15
// create array of random ints, then copy it
16
int
[] array1 = random.ints(
15_000_000
).toArray();
17
int
[] array2 =
new int
[array1.length];
18
System.arraycopy(array1,
0
, array2,
0
, array1.length);
19
20
// time the sorting of array1 with Arrays method sort
21
System.out.println(
"Starting sort"
);
22
Instant sortStart = Instant.now();
Arrays.sort(array1);
Instant sortEnd = Instant.now();
23
24
25
26
// display timing results
27
long
sortTime = Duration.between(sortStart, sortEnd).toMillis();
28
System.out.printf(
"Total time in milliseconds: %d%n%n"
, sortTime);
29
30
// time the sorting of array2 with Arrays method parallelSort
31
System.out.println(
"Starting parallelSort"
);
32
Instant parallelSortStart = Instant.now();
Fig. 23.28
|
Comparing performance of
Arrays
methods
sort
and
parallelSort
. (Part 1 of 2.)
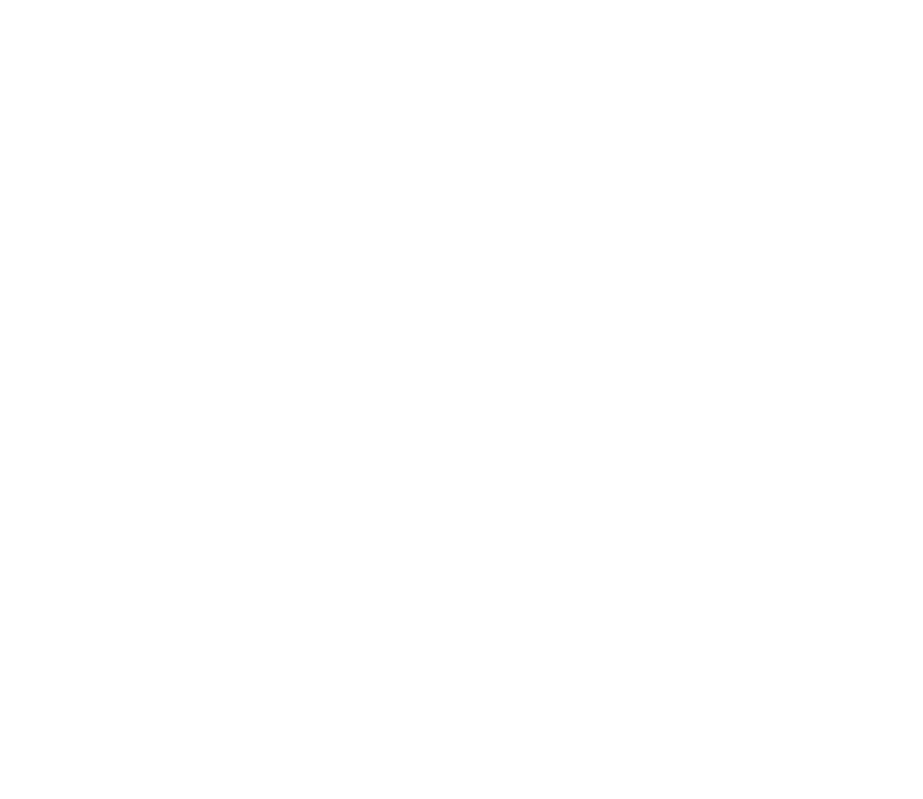













