Java Reference
In-Depth Information
32
33
// return value from buffer
34
public
synchronized
int
blockingGet()
throws
InterruptedException
35
{
36
// wait until buffer has data, then read value;
37
// while no data to read, place thread in waiting state
38
while
(occupiedCells ==
0
)
39
{
40
System.out.printf(
"Buffer is empty. Consumer waits.%n")
;
41
wait();
// wait until a buffer cell is filled
42
}
// end while
43
44
int
readValue = buffer[readIndex];
// read value from buffer
45
46
// update circular read index
47
readIndex = (readIndex +
1
) % buffer.length;
48
49
--occupiedCells;
// one fewer buffer cells are occupied
50
displayState(
"Consumer reads "
+ readValue);
51
notifyAll();
// notify threads waiting to write to buffer
52
53
return
readValue;
54
}
55
56
// display current operation and buffer state
57
public
synchronized
void
displayState(String operation)
58
{
59
// output operation and number of occupied buffer cells
60
System.out.printf(
"%s%s%d)%n%s"
, operation,
61
" (buffer cells occupied: "
, occupiedCells,
"buffer cells: "
);
62
63
for
(
int
value : buffer)
64
System.out.printf(
" %2d "
, value);
// output values in buffer
65
66
System.out.printf(
"%n "
);
67
68
for
(
int
i = 0; i < buffer.length; i++)
69
System.out.print(
"---- "
);
70
71
System.out.printf(
"%n "
);
72
73
for
(
int
i =
0
; i < buffer.length; i++)
74
{
75
if
(i == writeIndex && i == readIndex)
76
System.out.print(
" WR"
);
// both write and read index
77
else
if
(i == writeIndex)
78
System.out.print(
" W "
);
// just write index
79
else
if
(i == readIndex)
80
System.out.print(
" R "
);
// just read index
81
else
82
System.out.print(
" "
);
// neither index
83
}
84
Fig. 23.18
|
Synchronizing access to a shared three-element bounded buffer. (Part 2 of 3.)
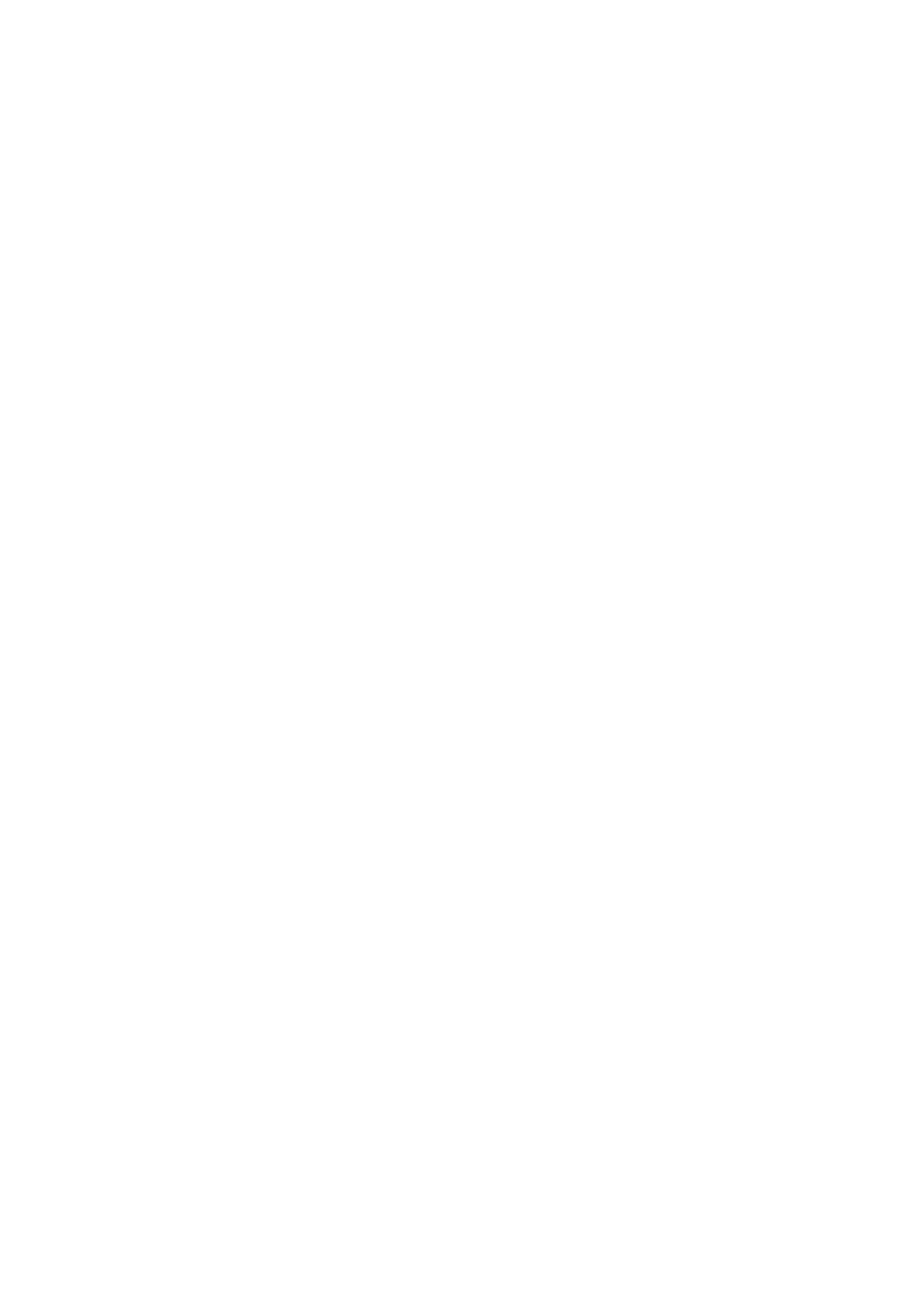



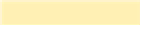

