Java Reference
In-Depth Information
Queue
object's
take
method (line 25). This method call
blocks
if necessary until there's an
element in the
buffer
to remove. Lines 18-19 and 26-27 use the
ArrayBlockingQueue
object's
size
method to display the total number of elements currently in the
Array-
BlockingQueue
.
Class
BlockingBufferTest
Class
BlockingBufferTest
(Fig. 23.15) contains the
main
method that launches the ap-
plication. Line 13 creates an
ExecutorService
, and line 16 creates a
BlockingBuffer
ob-
ject and assigns its reference to the
Buffer
variable
sharedLocation
. Lines 18-19 execute
the
Producer
and
Consumer
Runnable
s. Line 21 calls method
shutdown
to end the appli-
cation when the threads finish executing the
Producer
and
Consumer
tasks and line 22
waits for the scheduled tasks to complete.
1
// Fig. 23.15: BlockingBufferTest.java
2
// Two threads manipulating a blocking buffer that properly
3
// implements the producer/consumer relationship.
4
import
java.util.concurrent.ExecutorService;
5
import
java.util.concurrent.Executors;
6
import
java.util.concurrent.TimeUnit;
7
8
public
class
BlockingBufferTest
9
{
10
public
static
void
main(String[] args)
throws
InterruptedException
11
{
12
// create new thread pool with two threads
13
ExecutorService executorService = Executors.newCachedThreadPool();
14
15
// create BlockingBuffer to store ints
Buffer sharedLocation =
new
BlockingBuffer();
16
17
18
executorService.execute(
new
Producer(sharedLocation));
executorService.execute(
new
Consumer(sharedLocation));
19
20
21
executorService.shutdown();
22
executorService.awaitTermination(
1
,
TimeUnit.MINUTES
);
23
}
24
}
// end class BlockingBufferTest
Producer writes 1 Buffer cells occupied: 1
Consumer reads 1 Buffer cells occupied: 0
Producer writes 2 Buffer cells occupied: 1
Consumer reads 2 Buffer cells occupied: 0
Producer writes 3 Buffer cells occupied: 1
Consumer reads 3 Buffer cells occupied: 0
Producer writes 4 Buffer cells occupied: 1
Consumer reads 4 Buffer cells occupied: 0
Producer writes 5 Buffer cells occupied: 1
Consumer reads 5 Buffer cells occupied: 0
Producer writes 6 Buffer cells occupied: 1
Fig. 23.15
|
Two threads manipulating a blocking buffer that properly implements the
producer/consumer relationship. (Part 1 of 2.)
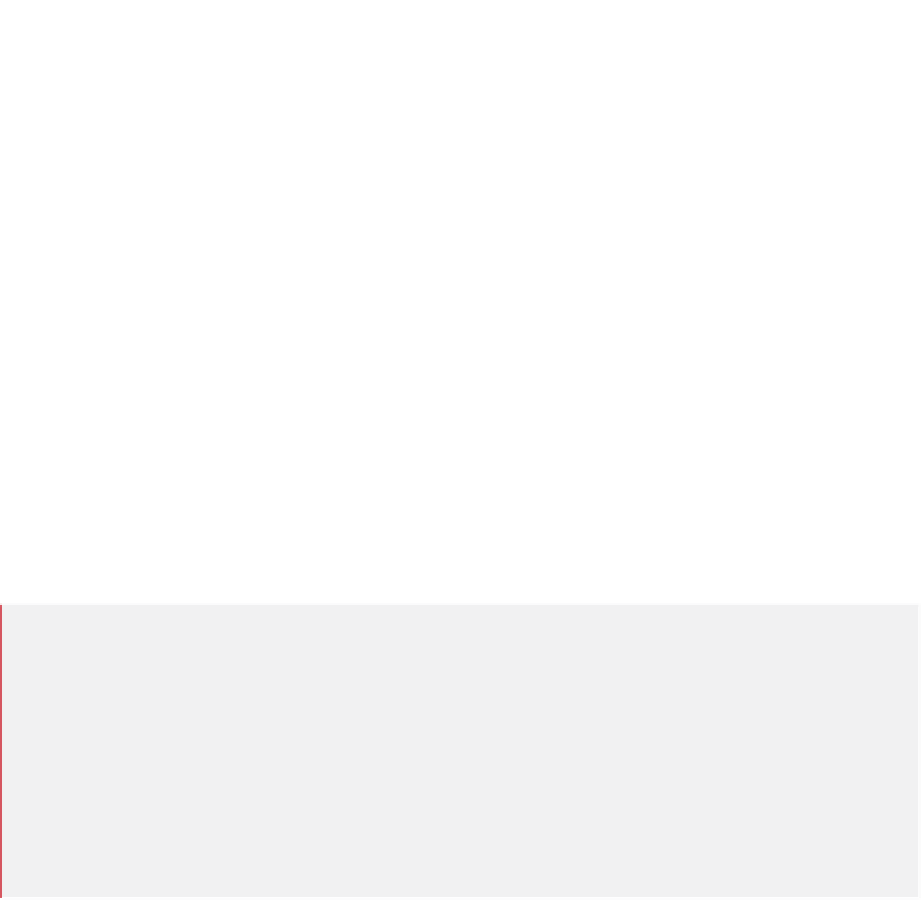















