Java Reference
In-Depth Information
Summary
Section 2.2 Your First Program in Java: Printing a Line of Text
• A Java application (p. 35) executes when you use the
java
command to launch the JVM.
• Comments (p. 36) document programs and improve their readability. The compiler ignores them.
• A comment that begins with
//
is an end-of-line comment—it terminates at the end of the line
on which it appears.
• Traditional comments (p. 36) can be spread over several lines and are delimited by
/*
and
*/
.
• Javadoc comments (p. 36), delimited by
/**
and
*/
, enable you to embed program documentation
in your code. The
javadoc
utility program generates HTML pages based on these comments.
• A syntax error (p. 36; also called a compiler error, compile-time error or compilation error) oc-
curs when the compiler encounters code that violates Java's language rules. It's similar to a gram-
mar error in a natural language.
• Blank lines, space characters and tab characters are known as white space (p. 37). White space
makes programs easier to read and is ignored by the compiler.
• Keywords (p. 37) are reserved for use by Java and are always spelled with all lowercase letters.
•Keyword
class
(p. 37) introduces a class declaration.
• By convention, all class names in Java begin with a capital letter and capitalize the first letter of
each word they include (e.g.,
SampleClassName
).
• A Java class name is an identifier—a series of characters consisting of letters, digits, underscores
(
_
) and dollar signs (
$
) that does not begin with a digit and does not contain spaces.
• Java is case sensitive (p. 38)—that is, uppercase and lowercase letters are distinct.
• The body of every class declaration (p. 38) is delimited by braces,
{
and
}
.
•A
public
(p. 37) class declaration must be saved in a file with the same name as the class followed
by the “
.java
” file-name extension.
•Method
main
(p. 38) is the starting point of every Java application and must begin with
public static void
main(String[] args)
otherwise, the JVM will not execute the application.
• Methods perform tasks and return information when they complete them. Keyword
void
(p. 38)
indicates that a method will perform a task but return no information.
• Statements instruct the computer to perform actions.
• A string (p. 39) in double quotes is sometimes called a character string or a string literal.
• The standard output object (
System.out
; p. 39) displays characters in the command window.
•Method
System.out.println
(p. 39) displays its argument (p. 39) in the command window fol-
lowed by a newline character to position the output cursor to the beginning of the next line.
• You compile a program with the command
javac
. If the program contains no syntax errors, a
class file (p. 40) containing the Java bytecodes that represent the application is created. These
bytecodes are interpreted by the JVM when you execute the program.
• To run an application, type
java
followed by the name of the class that contains the
main
method.
Section 2.3 Modifying Your First Java Program
•
System.out.print
(p. 42) displays its argument and positions the output cursor immediately af-
ter the last character displayed.
•A backslash (
\
) in a string is an escape character (p. 43). Java combines it with the next character to
form an escape sequence (p. 43). The escape sequence
\n
(p. 43) represents the newline character.
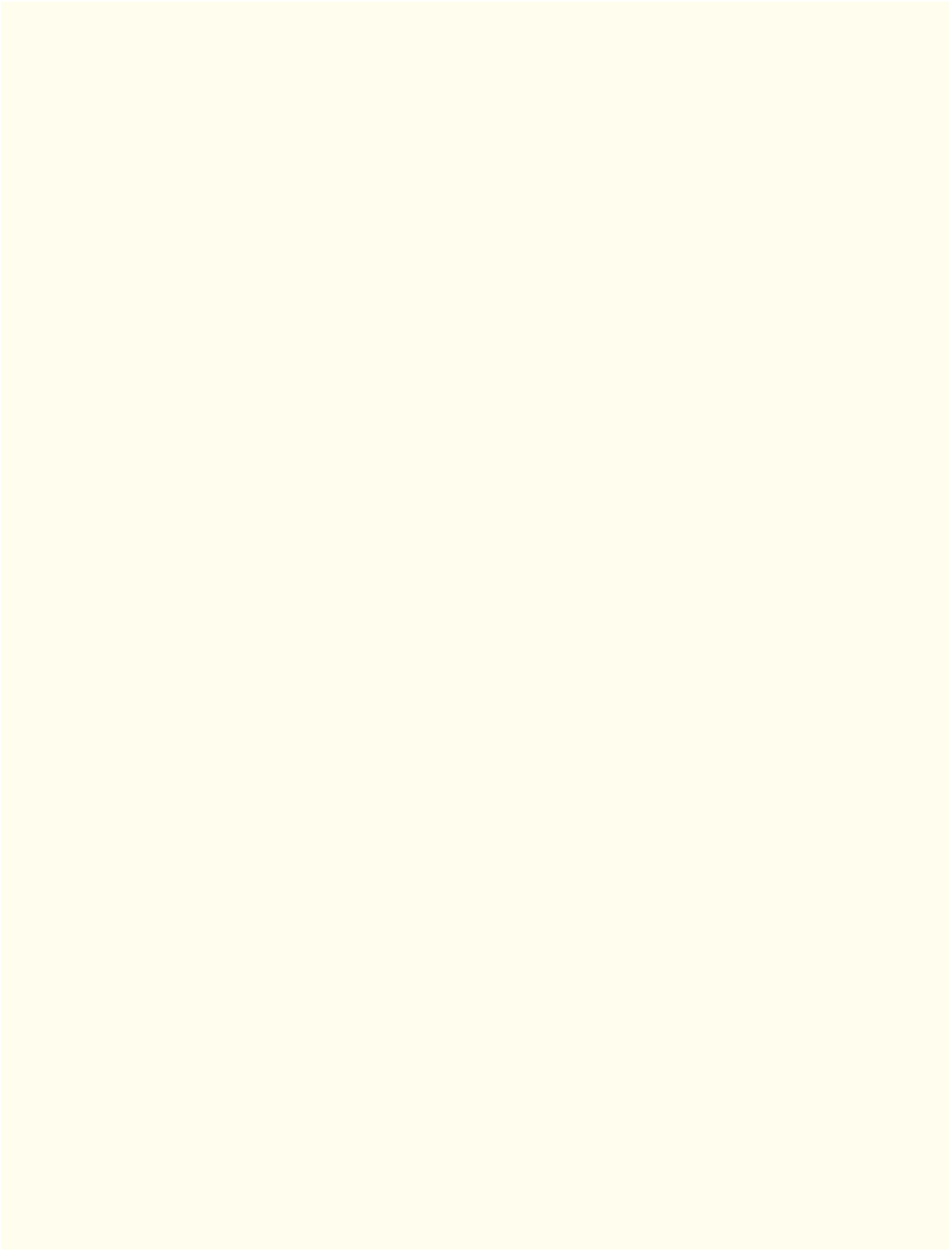