Java Reference
In-Depth Information
Using an
Executor
has many advantages over creating threads yourself.
Executor
s can
reuse existing threads
to eliminate the overhead of creating a new thread for each task and
can improve performance by
optimizing the number of threads
to ensure that the processor
stays busy, without creating so many threads that the application runs out of resources.
Software Engineering Observation 23.2
Though it's possible to create threads explicitly, it's recommended that you use the
Executor
interface to manage the execution of
Runnable
objects.
Using Class
Executors
to Obtain an
ExecutorService
The
ExecutorService
interface
(of package
java.util.concurrent
)
extends
Executor
and declares various methods for managing the life cycle of an
Executor
. You obtain an
ExecutorService
object by calling one of the
static
methods declared in class
Executors
(of package
java.util.concurrent
). We use interface
ExecutorService
and a method of
class
Executors
in our example, which executes three tasks.
Implementing the
Runnable
Interface
Class
PrintTask
(Fig. 23.3) implements
Runnable
(line 5),
so that multiple
PrintTask
s
can
execute concurrently
. Variable
sleepTime
(line 8) stores a random integer value from 0 to
5 seconds created in the
PrintTask
constructor (line 17). Each thread running a
Print-
Task
sleeps for the amount of time specified by
sleepTime
, then outputs its task's name
and a message indicating that it's done sleeping.
1
// Fig. 23.3: PrintTask.java
2
// PrintTask class sleeps for a random time from 0 to 5 seconds
3
import
java.security.SecureRandom;
4
5
public class
PrintTask
implements
Runnable
6
{
7
private static
final
SecureRandom generator =
new
SecureRandom();
8
private final int
sleepTime;
// random sleep time for thread
9
private final
String taskName;
10
11
// constructor
12
public
PrintTask(String taskName)
13
{
14
this
.taskName = taskName;
15
16
// pick random sleep time between 0 and 5 seconds
17
sleepTime = generator.nextInt(
5000
);
// milliseconds
18
}
19
20
// method run contains the code that a thread will execute
21
public void
run()
22
{
23
try
// put thread to sleep for sleepTime amount of time
24
{
25
System.out.printf(
"%s going to sleep for %d milliseconds.%n"
,
26
taskName, sleepTime);
Fig. 23.3
|
PrintTask
class sleeps for a random time from 0 to 5 seconds. (Part 1 of 2.)
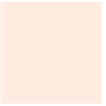












