Hardware Reference
In-Depth Information
Report It
To change the
variables in the HTML
documents, the server needs to read
those documents as it serves them. It
does this inside the
while()
block in the
sendFile()
method. Add the following
to that method. New lines are shown
in blue.
while (myFile.available()) {
// add the current char to the output string:
char thisChar = myFile.read();
outputString += thisChar;
// check for temperature variable and replace
// (floats can't be converted to Strings, so send it directly):
if (outputString.endsWith("$temperature")) {
outputString = "";
// limit the result to 2 decimal places:
thisClient.print(readSensor(),2);
}
When you run this, the server will read
the file. Whenever it hits one of the
named strings,
$temperature
,
$ther-
mostat
, or
$status
, it will replace that
string with the corresoponding vari-
able's value.
// check for thermostat variable and replace:
if (outputString.endsWith("$thermostat")) {
outputString.replace("$thermostat", String(thermostat));
}
Notice that the
if()
statement to
change the temperature is different
than the other two. There is no function
to convert a float variable to a String,
so you have to use
print()
or
println()
to do the job. That means you need to
print the temperature variable directly
to the client rather than just swapping
out that part of the string before you
print it.
// check for relay status variable and replace:
if (outputString.endsWith("$status")) {
String relayStatus = "off";
if (checkThermostat()) {
relayStatus = "on";
}
outputString.replace("$status", relayStatus);
}
// when you get a newline, send out and clear outputString:
if (thisChar == '\n') {
thisClient.print(outputString);
outputString = "";
}
}
Once you've made this change and uploaded
it, you're done. When you go to the Arduino's
address in your browser, you'll get the tem-
perature, the current thermostat setting, and the status of
the air conditioner. If you change any of the settings, you'll
change the temperature in your home as a result. With
the change you made to the image tag and the JavaScript
in the index.htm document to accommodate your camera,
you'll have an image from home as well. In a browser, your
final application should look like Figure 10-10.
popular mobile applications are developed by making
a basic browser shell without any user interface, then
developing the user interface in HTML5. When you click
through the app's interfaces, you're just browsing pages
on their site. Because so many mobile phone applica-
tions are mainly network applications, it makes sense to
use this as your primary approach. By doing so, you avail
yourself of all the protocols the Web has to offer.
The web approach to mobile apps means you get to take
advantage of protocols that don't run in a browser, but still
rely on HTTP. You'll see this in practice in the next project.
X
The great thing about using the browser as your appli-
cation interface for mobile applications is that you only
have to write the app once for all platforms. Many of the
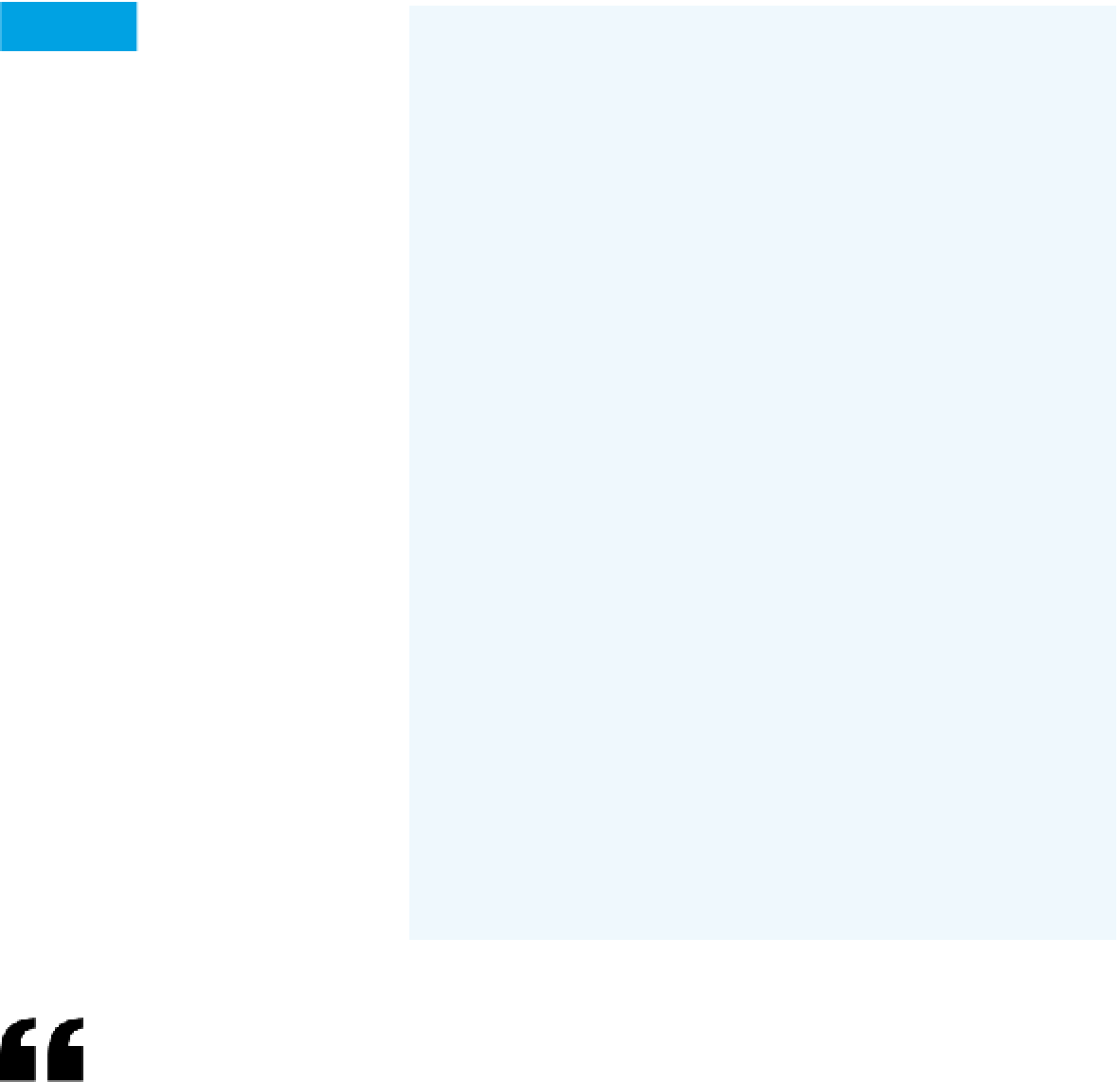



