Hardware Reference
In-Depth Information
The Code
Since the sketch is complex, it's built up piece-by-piece
below, as follows:
Make sure to install your circuit in an insulated box and
add plenty of strain relief to the AC lines. If they acci-
dentally disconnect, they can cause a short circuit—and
AC shorts are not pleasant. An electronic project case is
safest. You can add strain relief to the wires by using hot
glue or rubber caulking, though some cases come with
wire strain relief glands to hold the wire in place. Once
you've done that, give the wires a tug to make sure they
won't move. Take your time and get this part very secure
before you proceed.
1. Read the temperature sensor (for more on this sensor,
see Adafruit's tutorial at
www.ladyada.net/learn/
sensors/tmp36.html
).
2. Control the relay.
3. Read from the SD card.
4. Write a web server. This one will be more full-featured
than the one you wrote in Chapter 4.
Try It
Reading the temperature
sensor is pretty simple.
It outputs an analog voltage that's
proportional to the temperature. The
formula is:
/*
TMP36 Temperature reader and relay control
Context: Arduino
Reads a TMP36 temperature sensor
*/
Temp(°C) = (Vout (in mV) - 500) / 10
void setup() {
// initialize serial communication:
Serial.begin(9600);
}
Since you'll need to do other things in
the main loop, read the temperature
and convert it to Celsius in a separate
function. Return the result as a float.
void loop() {
Serial.print("Temperature: ");
Serial.println( readSensor() );
}
Your reading will look like this:
Temperature: 26.17
// read the temperature sensor:
float readSensor() {
// read the value from the sensor:
int sensorValue = analogRead(A0);
// convert the reading to volts:
float voltage = (sensorValue * 5.0) / 1024.0;
// convert the voltage to temperature in Celsius
// (100mv per degree - 500mV offset):
float temperature = (voltage - 0.5) * 100;
// return the temperature:
return temperature;
}
The reading is in Celsius, but if you
want to convert it to Fahrenheit, the
formula is:
F = 9/5*C + 32
When you run the sketch at this point,
you'll get a continual printout of the
temperature in the Serial Monitor.
Next, it's time to add the relay control.
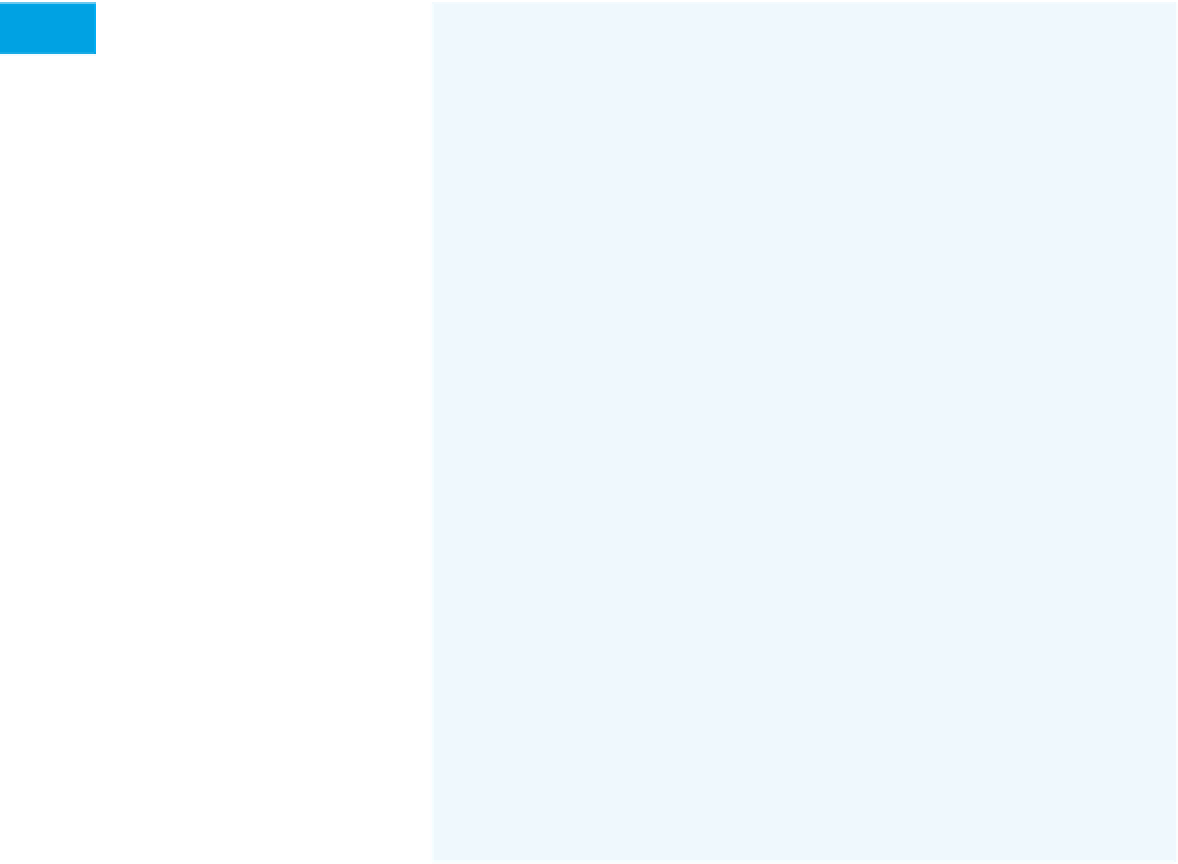


