Hardware Reference
In-Depth Information
The
serialEvent()
method gets any
incoming data as usual, and passes it
off to a method called
parseString()
.
That method splits the incoming string
into all the parts of the GPS sentence.
It passes the incoming sentences to a
few methods—
getRMC()
,
getGGA(),
and
getGSV(),
as appropriate—to
handle them. If you were writing a more
universal parser, you'd write similar
methods for each type of sentence.
8
void serialEvent(Serial myPort) {
// read the serial buffer:
String myString = myPort.readStringUntil('\n');
// if you got any bytes other than the linefeed, parse it:
if (myString != null) {
print(myString);
parseString(myString);
}
}
void parseString (String serialString) {
// split the string at the commas:
String items[] = (split(serialString, ','));
// if the first item in the sentence is the identifier, parse the rest
if (items[0].equals("$GPRMC")) {
// $GPRMC gives time, date, position, course, and speed
getRMC(items);
}
if (items[0].equals("$GPGGA")) {
// $GPGGA gives time, date, position, satellites used
getGGA(items);
}
if (items[0].equals("$GPGSV")) {
// $GPGSV gives satellites in view
satellitesInView = getGSV(items);
}
}
8
The
getRMC()
method converts
the latitude, longitude, and other
numerical parts of the sentence into
numbers.
void getRMC(String[] data) {
// move the items from the string into the variables:
int time = int(data[1]);
// first two digits of the time are hours:
hrs = time/10000;
// second two digits of the time are minutes:
mins = (time % 10000)/100;
// last two digits of the time are seconds:
secs = (time%100);
// if you have a valid reading, parse the rest of it:
if (data[2].equals("A")) {
latitude = minutesToDegrees(float(data[3]));
northSouth = data[4];
longitude = minutesToDegrees(float(data[5]));
eastWest = data[6];
heading = float(data[8]);
int date = int(data[9]);
»
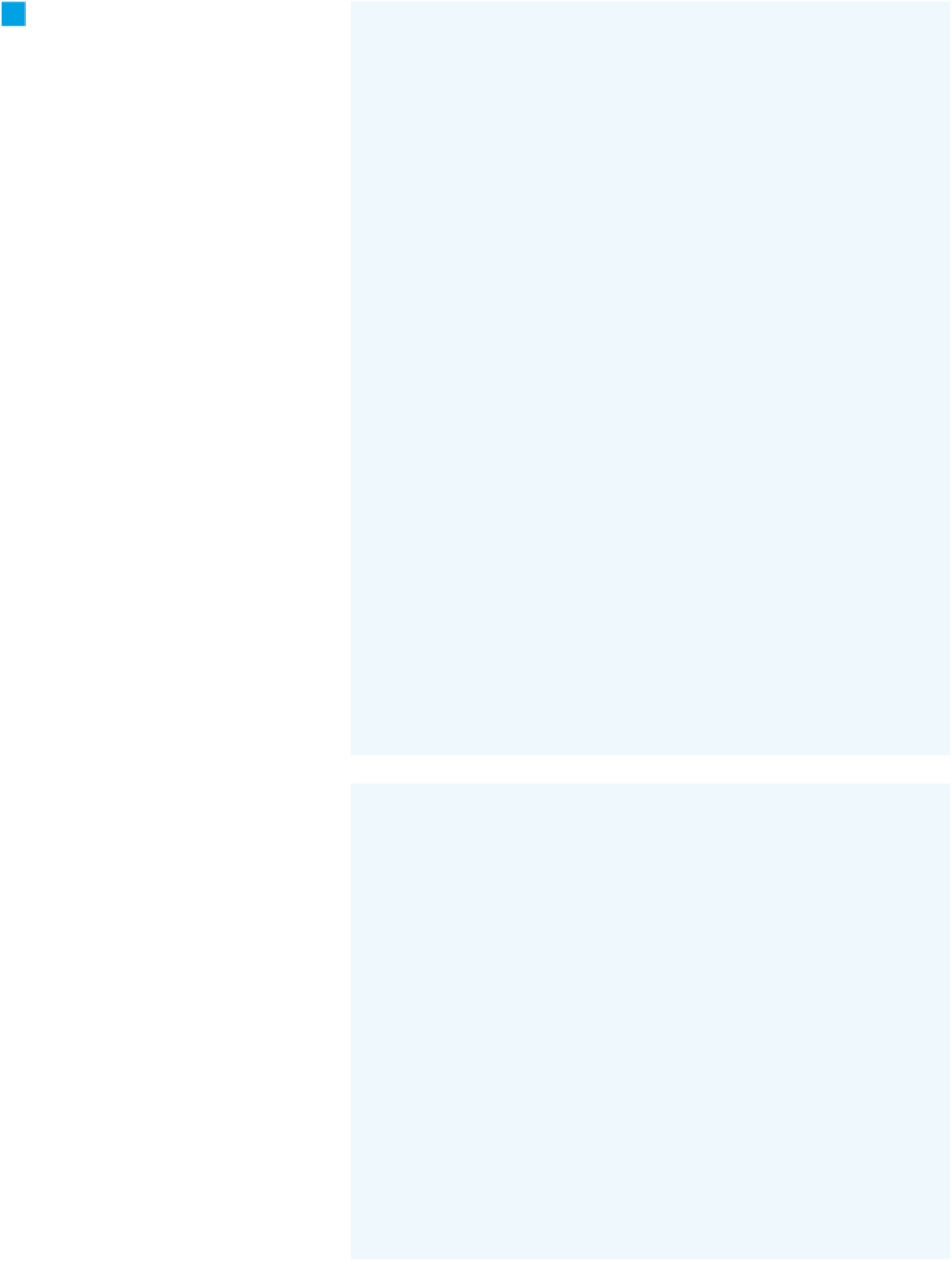



