Hardware Reference
In-Depth Information
Once the client's connected, it
sends an HTTP GET request.
Here's the
makeRequest()
method. It
returns a true value to set the
request-
ing
variable in the main loop, so the
client doesn't request twice while it's
connected.
8
boolean makeRequest() {
// make HTTP GET request and fill in the path to
// the PHP script on your server:
client.println("GET
/~myaccount/scraper.php
HTTP/1.1\n");
// fill in your server's name:
client.print("HOST:
example.com
\n\n");
// update the state of the program:
client.println();
return true;
}
8
Change these to
match your own server
name and path.
The server replies to
makeRequest()
like so:
HTTP/1.1 200 OK
Date: Fri, 14 Nov 2010 21:31:37 GMT
Server: Apache/2.0.52 (Red Hat)
Content-Length: 10
Connection: close
Content-Type: text/html; charset=UTF-8
Air Quality: 65
8
Once the client's found the air-
quality string and converted the bytes
that follow into an integer, it calls
setMeter()
to set the meter with the
result it obtained. You might want to
adjust the
AQIMax
value to reflect the
typical maximum for your geographic
area.
boolean setMeter(int thisLevel) {
Serial.println("setting meter...");
boolean result = false;
// map the result to a range the meter can use:
int meterSetting = map(thisLevel, 0, AQIMax, meterMin, meterMax);
// set the meter:
analogWrite(meterPin, meterSetting);
if (meterSetting > 0) {
result = true;
}
return result;
}
The last thing you need to do in
the main loop is set the indicator
LEDs so that you know where you are
in the program. You can use the
client.
connected()
status and the
meterIsSet
variable to set each of the LEDs.
8
void setLeds() {
// connected LED and disconnected LED can just use
// the client's connected() status:
digitalWrite(connectedLED, client.connected());
digitalWrite(disconnectedLED, !client.connected());
// success LED depends on reading being successful:
digitalWrite(successLED, meterIsSet);
}
That's the whole program. Once you've
got this program working on the micro-
controller, the controller will make
the HTTP GET request once every ten
minutes and set the meter accordingly.
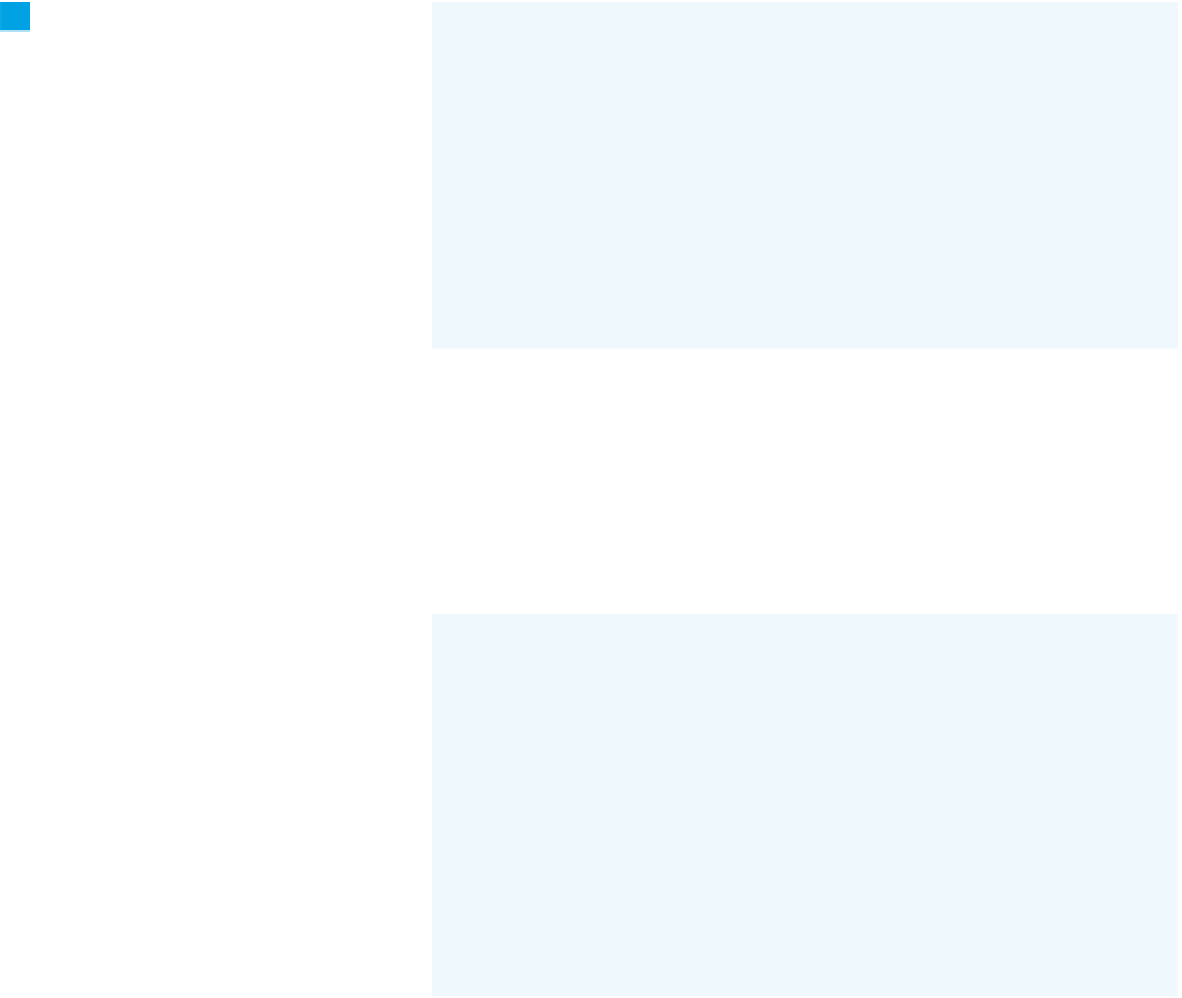




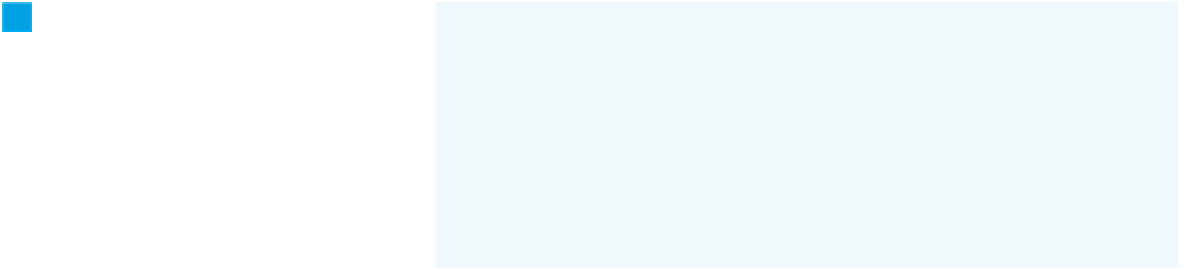





