Hardware Reference
In-Depth Information
Tame It
Every time the
program sends a mail
message, it should take note of the
time. Add a few new variables at the
beginning of the program.
int currentTime = 0; // the current time as a single number
int lastMailTime = 0; // last time you sent a mail
int mailInterval = 60; // minimum seconds between mails
String mailUrl = "http://www.example.com/cat-script.php";
8
Add one line at the beginning of
the
draw()
method to update
current-
Time
continuously.
void draw() {
currentTime = hour() * 3600 + minute() * 60 + second();
Now, modify the
sendMail()
method as shown in the code at
right.
8
void sendMail() {
// how long has passed since the last mail:
int timeDifference = currentTime - lastMailTime;
Once you're sure it works, adjust
mail-
Interval
to an appropriate minimum
number of seconds between emails.
if ( timeDifference > mailInterval) {
String[] mailScript = loadStrings(mailUrl);
println("results from mail script:");
println(mailScript);
// save the current minute for next time:
lastMailTime = currentTime;
}
}
When you run the sketch this time, you'll see an error message like this when you cross the threshold:
cat on mat
results from mail script:The file “http://www.example.com/cat-script.php” is missing or inaccessible, make sure the URL is
valid or that the file has been added to your sketch and is readable.
The sketch is now making an HTTP GET request to call a PHP script that's not there. In the next section, you'll write that
script.
Once you've confirmed that this script is running, go back
to the Processing sketch. Double-check that the
mailUrl
variable is the URL of the script, and run the sketch. When
the cat sits on the mat now, the sketch will call the mailer
script and you should get an email from the cat. The
console output will look like this:
Sending Mail from the Cat
Processing doesn't have any libraries for sending and
receiving email, so you can call a PHP script to do the
job. Processing has a simple command to make HTTP
GET requests:
loadStrings()
. The same technique can
be used to call other PHP scripts or web URLs from
Processing. POST requests are a little more complicated;
you'll see how to do that later.
cat on mat
results from mail script:
[0] “TO: you@example.com”
[1] “FROM: cat@example.com”
[2] “SUBJECT:the cat”
[3] “”
[4] “The cat is on the mat at http://www.example.com/
catcam.”
[5] “”
Save the following PHP script to your server with the
name cat-script.php. Test it from a browser. When you
call it, you should get an email in your inbox. Some mail
servers may require that you send mail only from your
proper account name. If that's the case, replace
cat@
example.com
with the email address for your account
name on the server on which the script is running.
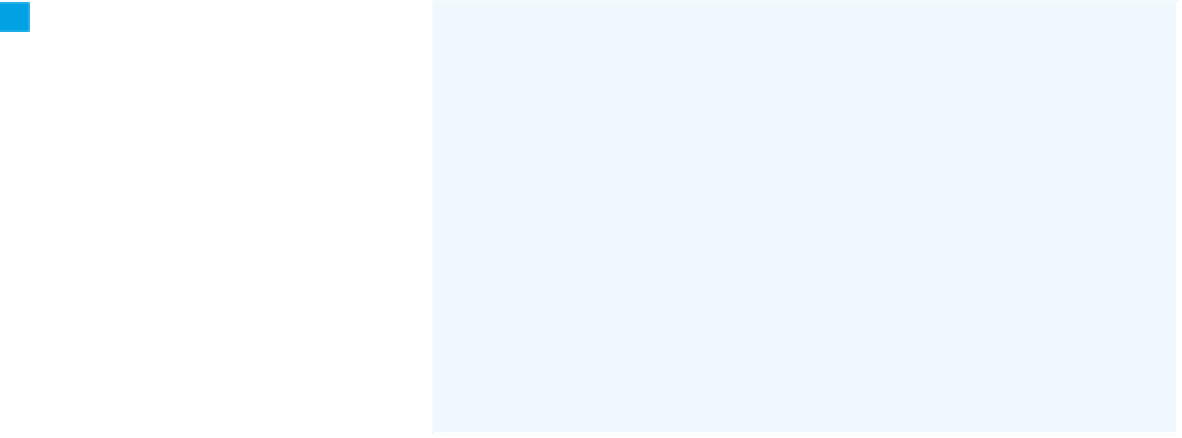

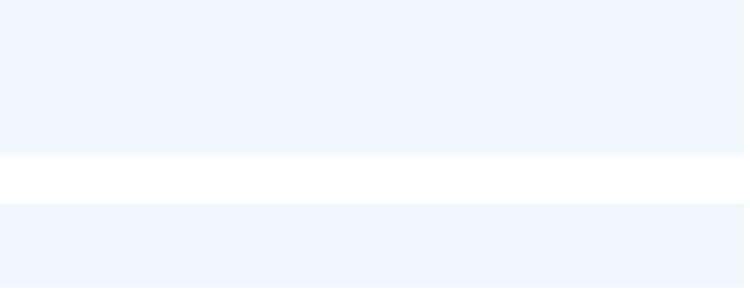





