Java Reference
In-Depth Information
80
public
Tree(Tree t1, Tree t2) {
81 root =
new
Node();
82 root.left = t1.root;
83 root.right = t2.root;
84 root.weight = t1.root.weight + t2.root.weight;
85 }
86
87
/** Create a tree containing a leaf node */
88
public
Tree(
int
weight,
char
element) {
89 root =
new
Node(weight, element);
90 }
91
92 @Override
/** Compare trees based on their weights */
93
public int
compareTo(Tree t) {
94
if
(root.weight < t.root.weight)
// Purposely reverse the order
95
return
1
;
96
else if
(root.weight == t.root.weight)
97
return
0
;
98
else
99
return
-1
;
100 }
101
102
public class
Node {
103
char
element;
// Stores the character for a leaf node
104
int
weight;
// weight of the subtree rooted at this node
105 Node left;
// Reference to the left subtree
106 Node right;
// Reference to the right subtree
107 String code =
""
;
// The code of this node from the root
108
109
/** Create an empty node */
110
public
Node() {
111 }
112
113
tree node
/** Create a node with the specified weight and character */
114
public
Node(
int
weight,
char
element) {
115
this
.weight = weight;
116
this
.element = element;
117 }
118 }
119 }
120 }
Enter text: Welcome
ASCII Code Character Frequency Code
87 W 1 110
99 c 1 111
101 e 2 10
108 l 1 011
109 m 1 010
111 o 1 00
The program prompts the user to enter a text string (lines 5-7) and counts the frequency
of the characters in the text (line 9). The
getCharacterFrequency
method (lines
66-73) creates an array
counts
to count the occurrences of each of the 256 ASCII char-
acters in the text. If a character appears in the text, its corresponding count is increased
by
1
(line 70).
getCharacterFrequency






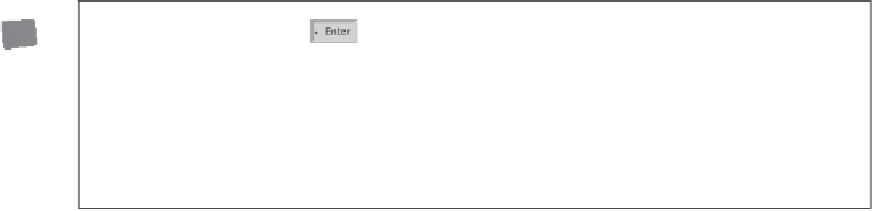
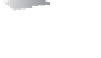






















Search WWH ::

Custom Search