Java Reference
In-Depth Information
Q
UIZ
P
ROGRAMMING
E
XERCISES
24.1
(
Add set operations in
MyList
) Define the following methods in
MyList
and
implement them in
MyAbstractList
:
/** Adds the elements in otherList to this list.
* Returns true if this list changed as a result of the call */
public boolean
addAll(MyList<E> otherList);
/** Removes all the elements in otherList from this list
* Returns true if this list changed as a result of the call */
public boolean
removeAll(MyList<E> otherList);
/** Retains the elements in this list that are also in otherList
* Returns true if this list changed as a result of the call */
public boolean
retainAll(MyList<E> otherList);
Write a test program that creates two
MyArrayList
s,
list1
and
list2
, with
the initial values
{"Tom", "George", "Peter", "Jean", "Jane"}
and
{"Tom", "George", "Michael", "Michelle", "Daniel"}
, then per-
form the following operations:
Invokes
list1.addAll(list2)
, and displays
list1
and
list2
.
■
Recreates
list1
and
list2
with the same initial values, invokes
list1.removeAll(list2)
, and displays
list1
and
list2
.
■
■
Recreates
list1
and
list2
with the same initial values, invokes
list1.retainAll(list2)
, and displays
list1
and
list2
.
*24.2
(
Implement
MyLinkedList
) The implementations of the methods
contains(E e)
,
get(int index)
,
indexOf(E e)
,
lastIndexOf(E e)
,
and
set(int index, E e)
are omitted in the text. Implement these methods.
*24.3
(
Implement a doubly linked list
) The
MyLinkedList
class used in Listing 24.6
is a one-way directional linked list that enables one-way traversal of the list.
Modify the
Node
class to add the new data field name
previous
to refer to the
previous node in the list, as follows:
public class
Node<E> {
E element;
Node<E> next;
Node<E> previous;
public
Node(E e) {
element = e;
}
}
Implement a new class named
TwoWayLinkedList
that uses a doubly
linked list to store elements. The
MyLinkedList
class in the text
extends
MyAbstractList
. Define
TwoWayLinkedList
to extend the
java.util.AbstractSequentialList
class. You need to implement all the
methods defined in
MyLinkedList
as well as the methods
listIterator()
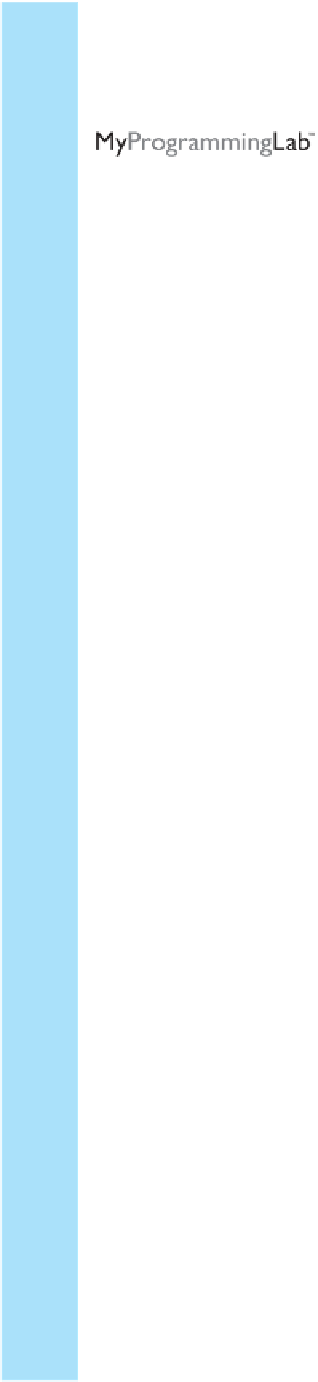

















Search WWH ::

Custom Search