Java Reference
In-Depth Information
17
new
TreeMap<>(hashMap);
18 System.out.println(
"Display entries in ascending order of key"
);
19 System.out.println(treeMap);
20
21
// Create a LinkedHashMap
22 Map<String, Integer> linkedHashMap =
23
new
LinkedHashMap<>(
16
,
0.75f
,
true
);
24 linkedHashMap.put(
"Smith"
,
30
);
25 linkedHashMap.put(
"Anderson"
,
31
);
26 linkedHashMap.put(
"Lewis"
,
29
);
27 linkedHashMap.put(
"Cook"
,
29
);
28
29
// Display the age for Lewis
30 System.out.println(
"\nThe age for "
+
"Lewis is "
+
31 linkedHashMap.get(
"Lewis"
));
32
33 System.out.println(
"Display entries in LinkedHashMap"
);
34 System.out.println(linkedHashMap);
35 }
36 }
linked hash map
Display entries in HashMap
{Cook=29, Smith=30, Lewis=29, Anderson=31}
Display entries in ascending order of key
{Anderson=31, Cook=29, Lewis=29, Smith=30}
The age for Lewis is 29
Display entries in LinkedHashMap
{Smith=30, Anderson=31, Cook=29, Lewis=29}
As shown in the output, the entries in the
HashMap
are in random order. The entries in the
TreeMap
are in increasing order of the keys. The entries in the
LinkedHashMap
are in the
order of their access, from least recently accessed to most recently.
All the concrete classes that implement the
Map
interface have at least two constructors.
One is the no-arg constructor that constructs an empty map, and the other constructs a map
from an instance of
Map
. Thus,
new TreeMap<String, Integer>(hashMap)
(lines 16-17)
constructs a tree map from a hash map.
You can create an insertion-ordered or access-ordered linked hash map. An access-ordered
linked hash map is created in lines 22-21. The most recently accessed entry is placed at the
end of the map. The entry with the key
Lewis
is last accessed in line 31, so it is displayed last
in line 34.
Tip
If you don't need to maintain an order in a map when updating it, use a
HashMap
.
When you need to maintain the insertion order or access order in the map, use a
LinkedHashMap
. When you need the map to be sorted on keys, use a
TreeMap
.
21.18
✓
✓
How do you create an instance of
Map
? How do you add an entry to a map consisting
of a key and a value? How do you remove an entry from a map? How do you find the
size of a map? How do you traverse entries in a map?
Check
Point
21.19
Describe and compare
HashMap
,
LinkedHashMap
, and
TreeMap
.



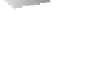






















Search WWH ::

Custom Search