Java Reference
In-Depth Information
retains the common elements in
set1
. Since
set1
and
set2
have no common elements,
set1
becomes empty.
21.2.2
LinkedHashSet
LinkedHashSet
extends
HashSet
with a linked-list implementation that supports an order-
ing of the elements in the set. The elements in a
HashSet
are not ordered, but the elements
in a
LinkedHashSet
can be retrieved in the order in which they were inserted into the set. A
LinkedHashSet
can be created by using one of its four constructors, as shown in FigureĀ 21.1.
These constructors are similar to the constructors for
HashSet
.
Listing 21.3 gives a test program for
LinkedHashSet
. The program simply replaces
HashSet
by
LinkedHashSet
in Listing 21.1.
linked hash set
L
ISTING
21.3
TestLinkedHashSet.java
1
import
java.util.*;
2
3
public class
TestLinkedHashSet {
4
public static void
main(String[] args) {
5
// Create a hash set
6
Set<String> set =
new
LinkedHashSet<>();
create linked hash set
7
8
// Add strings to the set
9 set.add(
"London"
);
10 set.add(
"Paris"
);
11 set.add(
"New York"
);
12 set.add(
"San Francisco"
);
13 set.add(
"Beijing"
);
14 set.add(
"New York"
);
15
16 System.out.println(set);
17
18
add element
// Display the elements in the hash set
19
for
(Object element: set)
display elements
20
System.out.print(element.toLowerCase() +
" "
);
21 }
22 }
[London, Paris, New York, San Francisco, Beijing]
london paris new york san francisco beijing
A
LinkedHashSet
is created in line 6. As shown in the output, the strings are stored in the order
in which they are inserted. Since
LinkedHashSet
is a set, it does not store duplicate elements.
The
LinkedHashSet
maintains the order in which the elements are inserted. To impose a
different order (e.g., increasing or decreasing order), you can use the
TreeSet
class, which is
introduced in the next section.
Tip
If you don't need to maintain the order in which the elements are inserted, use
HashSet
,
which is more efficient than
LinkedHashSet
.
21.2.3
TreeSet
SortedSet
is a subinterface of
Set
, which guarantees that the elements in the set are sorted.
Additionally, it provides the methods
first()
and
last()
for returning the first and last
elements in the set, and
headSet(toElement)
and
tailSet(fromElement)
for returning



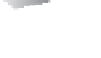






















Search WWH ::

Custom Search