Java Reference
In-Depth Information
java.util.Collections
+
sort(list: List): void
+
sort(list: List, c: Comparator): void
+
binarySearch(list: List, key: Object): int
+
binarySearch(list: List, key: Object, c:
Comparator): int
+
reverse(list: List): void
+
reverseOrder(): Comparator
+
shuffle(list: List): void
+
shuffle(list: List, rmd: Random): void
+
copy(des: List, src: List): void
+
nCopies(n: int, o: Object): List
+
fill(list: List, o: Object): void
+
max(c: Collection): Object
+
max(c: Collection, c: Comparator): Object
+
min(c: Collection): Object
+
min(c: Collection, c: Comparator): Object
+
disjoint(c1: Collection, c2: Collection):
boolean
+
frequency(c: Collection, o: Object): int
Sorts the specified list.
Sorts the specified list with the comparator.
Searches the key in the sorted list using binary search.
Searches the key in the sorted list using binary search
with the comparator.
Reverses the specified list.
Returns a comparator with the reverse ordering.
Shuffles the specified list randomly.
Shuffles the specified list with a random object.
Copies from the source list to the destination list.
Returns a list consisting of
n
copies of the object.
Fills the list with the object.
Returns the
max
object in the collection.
Returns the
max
object using the comparator.
Returns the
min
object in the collection.
Returns the
min
object using the comparator.
Returns true if
c1
and
c2
have no elements in common.
List
Collection
Returns the number of occurrences of the specified
element in the collection.
F
IGURE
20.7
The
Collections
class contains static methods for manipulating lists and collections.
You can sort the comparable elements in a list in its natural order with the
compareTo
method in the
Comparable
interface. You may also specify a comparator to sort elements.
For example, the following code sorts strings in a list.
sort list
List<String> list = Arrays.asList(
"red"
,
"green"
,
"blue"
);
Collections.sort(list);
System.out.println(list);
The output is
[blue, green, red]
.
The preceding code sorts a list in ascending order. To sort it in descending order, you can
simply use the
Collections.reverseOrder()
method to return a
Comparator
object
that orders the elements in reverse of their natural order. For example, the following code sorts
a list of strings in descending order.
ascending order
descending order
List<String> list = Arrays.asList(
"yellow"
,
"red"
,
"green"
,
"blue"
);
Collections.sort(list, Collections.reverseOrder());
System.out.println(list);
The output is
[yellow, red, green, blue]
.
You can use the
binarySearch
method to search for a key in a list. To use this method,
the list must be sorted in increasing order. If the key is not in the list, the method returns
-
binarySearch
1). Recall that the insertion point is where the item would fall in the list if
it were present. For example, the following code searches the keys in a list of integers and a
list of strings.
(
insertion point
+
List<Integer> list1 =
Arrays.asList(
2
,
4
,
7
,
10
,
11
,
45
,
50
,
59
,
60
,
66
);
System.out.println(
"(1) Index: "
+ Collections.binarySearch(list1,
7
));

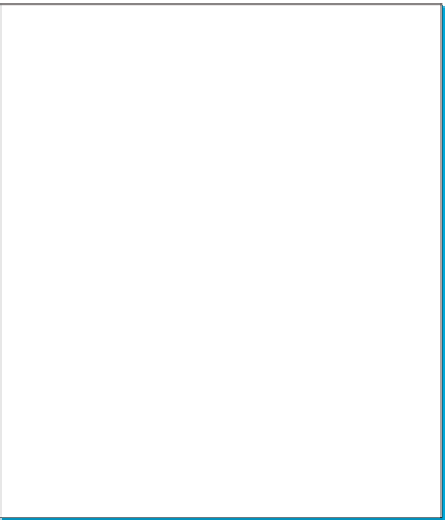


















Search WWH ::

Custom Search