Java Reference
In-Depth Information
5.
A generic class such as
ArrayList
used without a type parameter is called a
raw
type
. Use of raw types is allowed for backward compatibility with the earlier ver-
sions of Java.
6.
A wildcard generic type has three forms:
?
and
? extends T
, and
? super T
, where
T
is a generic type. The first form,
?
, called an
unbounded wildcard
, is the same as
? extends Object
. The second form,
? extends T
, called a
bounded wildcard
,
represents
T
or a subtype of
T
. The third form,
? super T
, called a
lower-bound wild-
card
, denotes
T
or a supertype of
T
.
7.
Generics are implemented using an approach called
type erasure
. The compiler uses
the generic type information to compile the code but erases it afterward, so the generic
information is not available at runtime. This approach enables the generic code to be
backward compatible with the legacy code that uses raw types.
8.
You cannot create an instance using a generic type parameter.
9.
You cannot create an array using a generic type parameter.
10.
You cannot use a generic type parameter of a class in a static context.
11.
Generic type parameters cannot be used in exception classes.
Q
UIZ
P
ROGRAMMING
E
XERCISES
19.1
(
Revising Listing 19.1
) Revise the
GenericStack
class in Listing 19.1 to imple-
ment it using an array rather than an
ArrayList
. You should check the array size
before adding a new element to the stack. If the array is full, create a new array that
doubles the current array size and copy the elements from the current array to the
new array.
19.2
(
Implement
GenericStack
using inheritance
) In Listing 19.1,
GenericStack
is
implemented using composition. Define a new stack class that extends
ArrayList
.
Draw the UML diagram for the classes and then implement
GenericStack
.
Write a test program that prompts the user to enter five strings and displays them in
reverse order.
19.3
(
Distinct elements in
ArrayList
) Write the following method that returns a new
ArrayList
. The new list contains the non-duplicate elements from the original list.
public static
<E> ArrayList<E> removeDuplicates(ArrayList<E> list)
19.4
(
Generic linear search
) Implement the following generic method for linear search.
public static
<E
extends
Comparable<E>>
int
linearSearch(E[] list, E key)
19.5
(
Maximum element in an array
) Implement the following method that returns the
maximum element in an array.
public static
<E
extends
Comparable<E>> E max(E[] list)
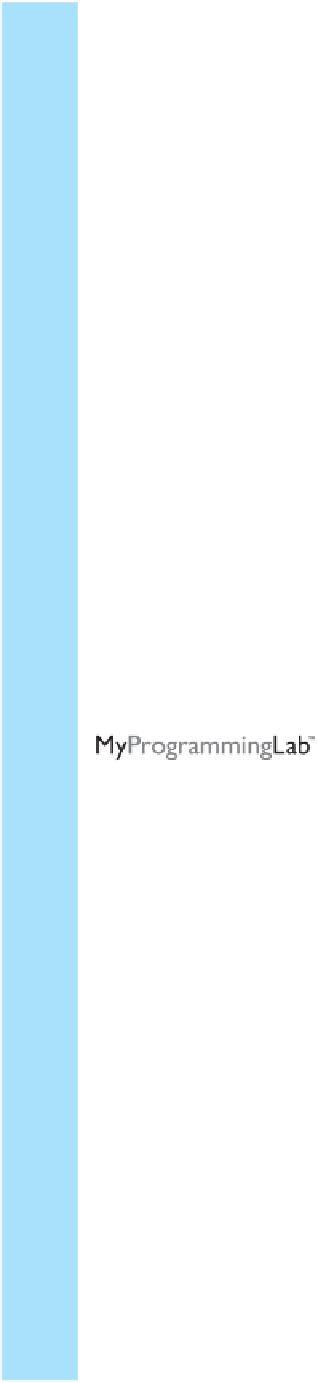

















Search WWH ::

Custom Search