Java Reference
In-Depth Information
a generic type parameter for a class in a static method, field, or initializer. For example, the
following code is illegal:
public class
Test<E> {
public static void
m(E o1) {
// Illegal
}
public static
E o1;
// Illegal
static
{
E o2;
// Illegal
}
}
Restriction 4: Exception Classes Cannot Be Generic
A generic class may not extend
java.lang.Throwable
, so the following class declara-
tion would be illegal:
public class
MyException<T>
extends
Exception {
}
Why? If it were allowed, you would have a
catch
clause for
MyException<T>
as follows:
try
{
...
}
catch
(MyException<T> ex) {
...
}
The JVM has to check the exception thrown from the
try
clause to see if it matches the
type specified in a
catch
clause. This is impossible, because the type information is not
present at runtime.
19.18
✓
✓
What is erasure? Why are Java generics implemented using erasure?
Check
19.19
Point
If your program uses
ArrayList<String>
and
ArrayList<Date>
, does the JVM
load both of them?
19.20
Can you create an instance using
new E()
for a generic type
E
? Why?
19.21
Can a method that uses a generic class parameter be static? Why?
19.22
Can you define a custom generic exception class? Why?
This section presents a case study on designing classes for matrix operations using
generic types.
The addition and multiplication operations for all matrices are similar except that their ele-
ment types differ. Therefore, you can design a superclass that describes the common opera-
tions shared by matrices of all types regardless of their element types, and you can define
subclasses tailored to specific types of matrices. This case study gives implementations for
two types:
int
and
Rational
. For the
int
type, the wrapper class
Integer
should be used
to wrap an
int
value into an object, so that the object is passed in the methods for operations.
The class diagram is shown in Figure 19.7. The methods
addMatrix
and
multiplyMatrix
add and multiply two matrices of a generic type
E[][]
. The static
method
printResult
displays the matrices, the operator, and their result. The methods
add
,
multiply
, and
zero
are abstract, because their implementations depend on the
specific type of the array elements. For example, the
zero()
method returns
0
for the
Key
Point


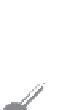


















Search WWH ::

Custom Search