Java Reference
In-Depth Information
A generic class or interface used without specifying a concrete type, called a raw type,
enables backward compatibility with earlier versions of Java.
Key
Point
You can use a generic class without specifying a concrete type like this:
GenericStack stack =
new
GenericStack();
// raw type
This is roughly equivalent to
GenericStack<Object> stack =
new
GenericStack<Object>();
A generic class such as
GenericStack
and
ArrayList
used without a type parameter is
called a
raw type
. Using raw types allows for backward compatibility with earlier versions of
Java. For example, a generic type has been used in
java.lang.Comparable
since JDK 1.5,
but a lot of code still uses the raw type
Comparable
, as shown in Listing 19.5:
raw type
backward compatibility
L
ISTING
19.5
Max.java
1
public class
Max {
2
/** Return the maximum of two objects */
3
public static
Comparable max(Comparable o1, Comparable o2) {
raw type
4
if
(o1.compareTo(o2) >
0
)
5
return
o1;
6
else
7
return
o2;
8 }
9 }
Comparable o1
and
Comparable o2
are raw type declarations. Be careful:
raw types are
unsafe
. For example, you might invoke the
max
method using
Max.max(
"Welcome"
,
23
);
// 23 is autoboxed into new Integer(23)
This would cause a runtime error, because you cannot compare a string with an inte-
ger object. The Java compiler displays a warning on line 3 when compiled with the option
-Xlint:unchecked
, as shown in FigureĀ 19.5.
Xlint:unchecked
F
IGURE
19.5
The unchecked warnings are displayed using the compiler option
-Xlint:unchecked
.
A better way to write the
max
method is to use a generic type, as shown in Listing 19.6.
L
ISTING
19.6
MaxUsingGenericType.java
1
public class
MaxUsingGenericType {
2
/** Return the maximum of two objects */
3
public static
<E
extends
Comparable<E>> E max(E o1, E o2) {
bounded type
4
if
(o1.compareTo(o2) >
0
)
5
return
o1;
6
else


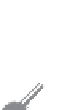

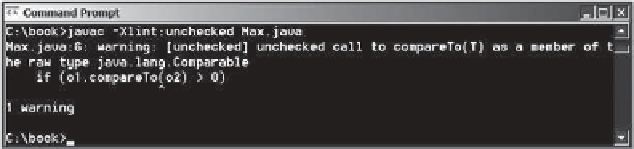

















Search WWH ::

Custom Search