Java Reference
In-Depth Information
This example creates a stack to hold integers and adds three integers to the stack:
GenericStack<Integer> stack2 =
new
GenericStack<>();
stack2.push(
1
);
// autoboxing 1 to new Integer(1)
stack2.push(
2
);
stack2.push(
3
);
Instead of using a generic type, you could simply make the type element
Object
, which can
accommodate any object type. However, using generic types can improve software reliability
and readability, because certain errors can be detected at compile time rather than at runtime.
For example, because
stack1
is declared
GenericStack<String>
, only strings can be
added to the stack. It would be a compile error if you attempted to add an integer to
stack1
.
benefits of using generic types
Caution
To create a stack of strings, you use
new GenericStack<String>()
or
new
GenericStack<>()
. This could mislead you into thinking that the constructor of
GenericStack
should be defined as
generic class constructor
public
GenericStack<E>()
This is wrong. It should be defined as
public
GenericStack()
Note
Occasionally, a generic class may have more than one parameter. In this case, place
the parameters together inside the brackets, separated by commas—for example,
<E1, E2, E3>
.
multiple generic parameters
Note
You can define a class or an interface as a subtype of a generic class or interface. For
example, the
java.lang.String
class is defined to implement the
Comparable
interface in the Java API as follows:
inheritance with generics
public class
String
implements
Comparable<String>
19.4
✓
✓
What is the generic definition for
java.lang.Comparable
in the Java API?
Check
19.5
Point
Since you create an instance of
ArrayList
of strings using
new
ArrayList<String>()
, should the constructor in the
ArrayList
class be
defined as
public
ArrayList<E>()
19.6
Can a generic class have multiple generic parameters?
19.7
How do you declare a generic type in a class?
A generic type can be defined for a static method.
Key
Point
You can define generic interfaces (e.g., the
Comparable
interface in Figure 19.1b) and
classes (e.g., the
GenericStack
class in Listing 19.1). You can also use generic types to
define generic methods. For example, Listing 19.2 defines a generic method
print
(lines
10-14) to print an array of objects. Line 6 passes an array of integer objects to invoke the
generic
print
method. Line 7 invokes
print
with an array of strings.
generic method





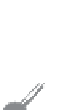


















Search WWH ::

Custom Search