Java Reference
In-Depth Information
The following statements create data streams. The first statement creates an input stream for
the file
in.dat
; the second statement creates an output stream for the file
out.dat
.
DataInputStream input =
new
DataInputStream(
new
FileInputStream(
"in.dat"
));
DataOutputStream output =
new
DataOutputStream(
new
FileOutputStream(
"out.dat"
));
Listing 17.2 writes student names and scores to a file named
temp.dat
and reads the data back
from the file.
L
ISTING
17.2
TestDataStream.java
1
import
java.io.*;
2
3
public class
TestDataStream {
4
public static void
main(String[] args)
throws
IOException {
5
try
(
// Create an output stream for file temp.dat
6
DataOutputStream output =
output stream
7
new
DataOutputStream(
new
FileOutputStream(
"temp.dat"
));
8 ) {
9
// Write student test scores to the file
10 output.writeUTF(
"John"
);
11 output.writeDouble(
85.5
);
12 output.writeUTF(
"Jim"
);
13 output.writeDouble(
185.5
);
14 output.writeUTF(
"George"
);
15 output.writeDouble(
105.25
);
16 }
17
18
output
try
(
// Create an input stream for file temp.dat
19
DataInputStream input =
input stream
20
new
DataInputStream(
new
FileInputStream(
"temp.dat"
));
21 ) {
22
// Read student test scores from the file
23 System.out.println(input.readUTF() +
" "
+ input.readDouble());
24 System.out.println(input.readUTF() +
" "
+ input.readDouble());
25 System.out.println(input.readUTF() +
" "
+ input.readDouble());
26 }
27 }
28 }
input
John 85.5
Susan 185.5
Kim 105.25
A
DataOutputStream
is created for file
temp.dat
in lines 6 and 7. Student names and scores
are written to the file in lines 10-15. A
DataInputStream
is created for the same file in lines
19-20. Student names and scores are read back from the file and displayed on the console in
lines 23-25.
DataInputStream
and
DataOutputStream
read and write Java primitive-type values
and strings in a machine-independent fashion, thereby enabling you to write a data file on one
machine and read it on another machine that has a different operating system or file structure.
An application uses a data output stream to write data that can later be read by a program using
a data input stream.
DataInputStream
filters data from an input stream into appropriate primitive-type val-
ues or strings.
DataOutputStream
converts primitive-type values or strings into bytes and









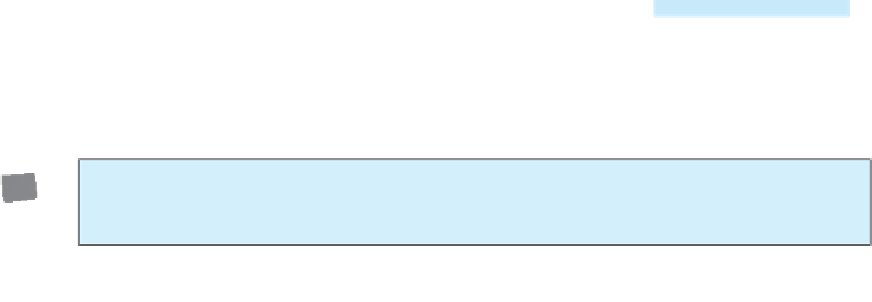
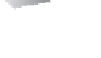





















Search WWH ::

Custom Search