Java Reference
In-Depth Information
Here are examples for reading values of various types from the keyboard:
1 Scanner input =
new
Scanner(System.in);
2 System.out.print(
"Enter a byte value: "
);
3
byte
byteValue = input.nextByte();
4
5 System.out.print(
"Enter a short value: "
);
6
short
shortValue = input.nextShort();
7
8 System.out.print(
"Enter an int value: "
);
9
int
intValue = input.nextInt();
10
11 System.out.print(
"Enter a long value: "
);
12
long
longValue = input.nextLong();
13
14 System.out.print(
"Enter a float value: "
);
15
float
floatValue = input.nextFloat();
If you enter a value with an incorrect range or format, a runtime error would occur. For
example, you enter a value
128
for line 3, an error would occur because
128
is out of range
for a
byte
type integer.
2.9.3 Numeric Operators
The operators for numeric data types include the standard arithmetic operators: addition (
+
),
subtraction (
-
), multiplication (
*
), division (
/
), and remainder (
%
), as shown in Table 2.3. The
operands
are the values operated by an operator.
operators
+
,
-
,
*
,
/
,
%
operands
T
ABLE
2.3
Numeric Operators
Name
Meaning
Example
Result
Addition
34
+
1
35
+
-
Subtraction
34.0 - 0.1
33.9
*
Multiplication
300 * 30
9000
/
Division
1.0 / 2.0
0.5
Remainder
20 % 3
2
%
When both operands of a division are integers, the result of the division is the quotient
and the fractional part is truncated. For example,
5 / 2
yields
2
, not
2.5
, and
-5 / 2
yields
-2
, not
-2.5
. To perform a float-point division, one of the operands must be a floating-point
number. For example,
5.0 / 2
yields
2.5
.
The
%
operator, known as
remainder
or
modulo
operator, yields the remainder after divi-
sion. The operand on the left is the dividend and the operand on the right is the divisor. There-
fore,
7 % 3
yields
1
,
3 % 7
yields
3
,
12 % 4
yields
0
,
26 % 8
yields
2
, and
20 % 13
yields
7
.
integer division
2
0
3
3
1
Quotient
Divisor
37
6
1
73
0
3
4 2
12
0
8 6
24
2
13
20
13
7
Dividend
Remainder
The
%
operator is often used for positive integers, but it can also be used with negative inte-
gers and floating-point values. The remainder is negative only if the dividend is negative. For
example,
-7 % 3
yields
-1
,
-12 % 4
yields
0
,
-26 % -8
yields
-2
, and
20 % -13
yields
7
.
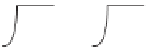





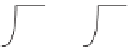
























Search WWH ::

Custom Search