Java Reference
In-Depth Information
The code in lines 35-41 can be replaced by using binding properties as follows:
text.xProperty().bind(slHorizontal.valueProperty().
multiply(paneForText.widthProperty()).
divide(slHorizontal.maxProperty()));
text.yProperty().bind((slVertical.maxProperty().subtract(
slVertical.valueProperty()).multiply(
paneForText.heightProperty().divide(
slVertical.maxProperty()))));
Listing 15.17 gives a program that displays a bouncing ball. You can add a slider to control
the speed of the ball movement as shown in FigureĀ 16.26. The new program is given in
Listing 16.12.
F
IGURE
16.26
You can increase or decrease the speed of the ball using a slider.
L
ISTING
16.12
BounceBallSlider.java
1
import
javafx.application.Application;
2
import
javafx.stage.Stage;
3
import
javafx.scene.Scene;
4
import
javafx.scene.control.Slider;
5
import
javafx.scene.layout.BorderPane;
6
7
public class
BounceBallSlider
extends
Application {
8 @Override
// Override the start method in the Application class
9
public void
start(Stage primaryStage) {
10 BallPane ballPane =
new
BallPane();
11 Slider slSpeed =
new
Slider();
12 slSpeed.setMax(
20
);
13
create a ball pane
create a slider
set max value for slider
bind rate with slider value
ballPane.rateProperty().bind(slSpeed.valueProperty());
14
15 BorderPane pane =
new
BorderPane();
16 pane.setCenter(ballPane);
17 pane.setBottom(slSpeed);
18
19
// Create a scene and place it in the stage
20 Scene scene =
new
Scene(pane,
250
,
250
);
21 primaryStage.setTitle(
"BounceBallSlider"
);
// Set the stage title
22 primaryStage.setScene(scene);
// Place the scene in the stage
23 primaryStage.show();
// Display the stage
24 }
25 }
create a border pane
add ball pane to center
add slider to the bottom
The
BallPane
class defined in Listing 15.17 animates a ball bouncing in a pane.
TheĀ
rateProperty()
method in
BallPane
returns a property value for animation rate.

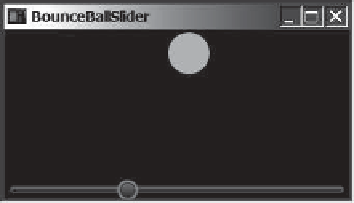

















Search WWH ::

Custom Search