Java Reference
In-Depth Information
4
import
javafx.scene.text.Text;
5
import
javafx.stage.Stage;
6
7
public class
MouseEventDemo
extends
Application {
8 @Override
// Override the start method in the Application class
9
public void
start(Stage primaryStage) {
10
// Create a pane and set its properties
11 Pane pane =
new
Pane();
12 Text text =
new
Text(
20
,
20
,
"Programming is fun"
);
13 pane.getChildren().addAll(text);
14
create a pane
create a text
add text to a pane
lambda handler
reset text position
text.setOnMouseDragged(e -> {
15
text.setX(e.getX());
16
text.setY(e.getY());
17
});
18
19
// Create a scene and place it in the stage
20 Scene scene =
new
Scene(pane,
300
,
100
);
21 primaryStage.setTitle(
"MouseEventDemo"
);
// Set the stage title
22 primaryStage.setScene(scene);
// Place the scene in the stage
23 primaryStage.show();
// Display the stage
24 }
25 }
Each node or scene can fire mouse events. The program creates a
Text
(line 12) and registers
a handler to handle move dragged event (line 14). Whenever a mouse is dragged, the text's
x
- and
y
-coordinates are set to the mouse position (lines 15 and 16).
15.14
✓
✓
What method do you use to get the mouse-point position for a mouse event?
Check
15.15
What methods do you use to register a handler for a mouse pressed, released, clicked,
entered, exited, moved and dragged event?
Point
A
KeyEvent
is fired whenever a key is pressed, released, or typed on a node or a scene.
Key events
enable the use of the keys to control and perform actions or get input from the
keyboard. The
KeyEvent
object describes the nature of the event (namely, that a key has been
pressed, released, or typed) and the value of the key, as shown in Figure 15.12.
Key
Point
javafx.scene.input.KeyEvent
+getCharacter(): String
+getCode(): KeyCode
+getText(): String
+isAltDown(): boolean
+isControlDown(): boolean
+isMetaDown(): boolean
+isShiftDown(): boolean
Returns the character associated with the key in this event.
Returns the key code associated with the key in this event.
Returns a string describing the key code.
Returns true if the
Alt
key is pressed on this event.
Returns true if the
Control
key is pressed on this event.
Returns true if the mouse
Meta
button is pressed on this event.
Returns true if the
Shift
key is pressed on this event.
F
IGURE
15.12
The
KeyEvent
class encapsulates information about key events.
Every key event has an associated code that is returned by the
getCode()
method in
KeyEvent
. The
key codes
are constants defined in
KeyCode
. Table 15.2 lists some constants.
KeyCode
is an
enum
type. For use of
enum
types, see Appendix I. For the key-pressed and






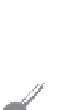


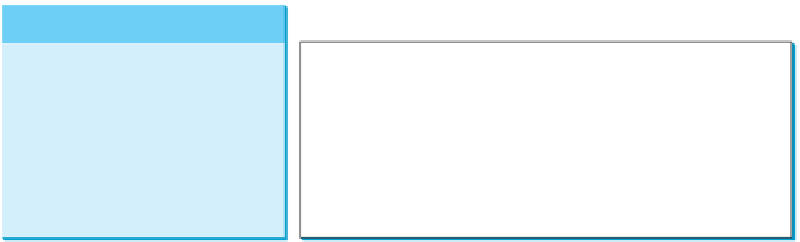
















Search WWH ::

Custom Search