Java Reference
In-Depth Information
L
ISTING
14.3
ButtonInPane.java
1
import
javafx.application.Application;
2
import
javafx.scene.Scene;
3
import
javafx.scene.control.Button;
4
import
javafx.stage.Stage;
5
import
javafx.scene.layout.StackPane;
6
7
public class
ButtonInPane
extends
Application {
8 @Override
// Override the start method in the Application class
9
public void
start(Stage primaryStage) {
10
// Create a scene and place a button in the scene
11 StackPane pane =
new
StackPane();
12 pane.getChildren().add(
new
Button(
"
OK
"
));
13 Scene scene =
new
Scene(pane,
200
,
50
);
14 primaryStage.setTitle(
"
Button in a pane
"
);
// Set the stage title
15 primaryStage.setScene(scene);
// Place the scene in the stage
16
create a pane
add a button
add pane to scene
primaryStage.show();
// Display the stage
display stage
17 }
18 }
main method omitted
F
IGURE
14.4
A button is placed in the center of the pane.
The program creates a
StackPane
(line 11) and adds a button as a child of the pane (line 12).
The
getChildren()
method returns an instance of
javafx.collections.ObservableList
.
ObservableList
behaves very much like an
ArrayList
for storing a collection of elements.
Invoking
add(e)
adds an element to the list. The
StackPane
places the nodes in the center
of the pane on top of each other. Here, there is only one node in the pane. The
StackPane
respects a node's preferred size. So you see the button displayed in its preferred size.
Listing 14.4 gives an example that displays a circle in the center of the pane, as shown in
FigureĀ 14.5a.
ObservableList
L
ISTING
14.4
ShowCircle.java
1
import
javafx.application.Application;
2
import
javafx.scene.Scene;
3
import
javafx.scene.layout.Pane;
4
import
javafx.scene.paint.Color;
5
import
javafx.scene.shape.Circle;
6
import
javafx.stage.Stage;
7
8
public class
ShowCircle
extends
Application {
9 @Override
// Override the start method in the Application class
10
public void
start(Stage primaryStage) {
11
// Create a circle and set its properties
12 Circle circle =
new
Circle();
13 circle.setCenterX(
100
);
14 circle.setCenterY(
100
);
15 circle.setRadius(
50
);
16 circle.setStroke(Color.BLACK);
17 circle.setFill(Color.WHITE);
18
19
create a circle
set circle properties
// Create a pane to hold the circle
20
Pane pane =
new
Pane();
create a pane




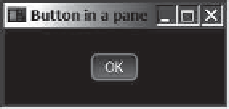


















Search WWH ::

Custom Search