Java Reference
In-Depth Information
10
11
// Read data from a file
12
while
(input.hasNext()) {
13 String firstName = input.next();
14 String mi = input.next();
15 String lastName = input.next();
16
int
score = input.nextInt();
17 System.out.println(
18 firstName +
""
+ mi +
""
+ lastName +
""
+ score);
19
scores.txt
has next?
read items
John T Smith 90
Eric K Jones 85
}
20
21
// Close the file
22 input.close();
23
close file
}
24 }
Note that
new Scanner(String)
creates a
Scanner
for a given string. To create a
Scanner
to read data from a file, you have to use the
java.io.File
class to create an instance of the
File
using the constructor
new File(filename)
(line 6), and use
new Scanner(File)
to create a
Scanner
for the file (line 9).
Invoking the constructor
new Scanner(File)
may throw an I/O exception, so the
main
method declares
throws Exception
in line 4.
Each iteration in the
while
loop reads the first name, middle initial, last name, and score
from the text file (lines 12-19). The file is closed in line 22.
It is not necessary to close the input file (line 22), but it is a good practice to do so to release
the resources occupied by the file. You can rewrite this program using the try-with-resources
File
class
throws Exception
close file
12.11.4 How Does
Scanner
Work?
The
nextByte()
,
nextShort()
,
nextInt()
,
nextLong()
,
nextFloat()
,
next-
Double()
, and
next()
methods are known as
token-reading methods
, because they read
tokens separated by delimiters. By default, the delimiters are whitespace characters. You
can use the
useDelimiter(String regex)
method to set a new pattern for delimiters.
How does an input method work? A token-reading method first skips any delimiters (whites-
pace characters by default), then reads a token ending at a delimiter. The token is then auto-
matically converted into a value of the
byte
,
short
,
int
,
long
,
float
, or
double
type for
nextByte()
,
nextShort()
,
nextInt()
,
nextLong()
,
nextFloat()
, and
nextDou-
ble()
, respectively. For the
next()
method, no conversion is performed. If the token does
not match the expected type, a runtime exception
java.util.InputMismatchException
will be thrown.
Both methods
next()
and
nextLine()
read a string. The
next()
method reads a string
delimited by delimiters, and
nextLine()
reads a line ending with a line separator.
token-reading method
change delimiter
InputMismatchException
next()
vs.
nextLine()
Note
The line-separator string is defined by the system. It is
\r\n
on Windows and
\n
on
UNIX. To get the line separator on a particular platform, use
line separator
String lineSeparator = System.getProperty(
"line.separator"
);
If you enter input from a keyboard, a line ends with the
Enter
key, which corresponds
to the
\n
character.
The token-reading method does not read the delimiter after the token. If the
nextLine()
method is invoked after a token-reading method, this method reads characters that start from
this delimiter and end with the line separator. The line separator is read, but it is not part of the
string returned by
nextLine()
.
behavior of
nextLine()


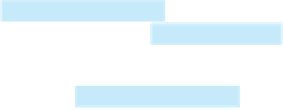


























Search WWH ::

Custom Search