Java Reference
In-Depth Information
try
{
...
}
catch
(Exception ex) {
...
}
catch
(RuntimeException ex) {
...
}
try
{
...
}
catch
(RuntimeException ex) {
...
}
catch
(Exception ex) {
...
}
(a) Wrong order
(b) Correct order
Note
Java forces you to deal with checked exceptions. If a method declares a checked exception
(i.e., an exception other than
Error
or
RuntimeException
), you must invoke it in a
try-catch
block or declare to throw the exception in the calling method. For example,
suppose that method
p1
invokes method
p2
, and
p2
may throw a checked exception
(e.g.,
IOException
); you have to write the code as shown in (a) or (b) below.
catch or declare checked
exceptions
void
p1() {
try
{
p2();
}
catch
(IOException ex)
{
...
}
void
p1()
throws
IOException {
p2();
}
}
(a) Catch exception
(b) Throw exception
Note
You can use the new JDK 7 multi-catch feature to simplify coding for the exceptions
with the same handling code. The syntax is:
JDK 7 multi-catch
catch
(Exception1 | Exception2 | ... | Exceptionk ex) {
// Same code for handling these exceptions
}
Each exception type is separated from the next with a vertical bar (
|
). If one of the
exceptions is caught, the handling code is executed.
12.4.4 Getting Information from Exceptions
An exception object contains valuable information about the exception. You may use the fol-
lowing instance methods in the
java.lang.Throwable
class to get information regarding
the exception, as shown in FigureĀ 12.4. The
printStackTrace()
method prints stack trace
methods in
Throwable
java.lang.Throwable
+getMessage(): String
+toString(): String
Returns the message that describes this exception object.
Returns the concatenation of three strings: (1) the full name of the exception
class; (2)
":"
(a colon and a space); (3) the
getMessage()
method.
Prints the
Throwable
object and its call stack trace information on the
console.
Returns an array of stack trace elements representing the stack trace
pertaining to this exception object.
+printStackTrace(): void
+getStackTrace():
StackTraceElement[]
F
IGURE
12.4
Throwable
is the root class for all exception objects.

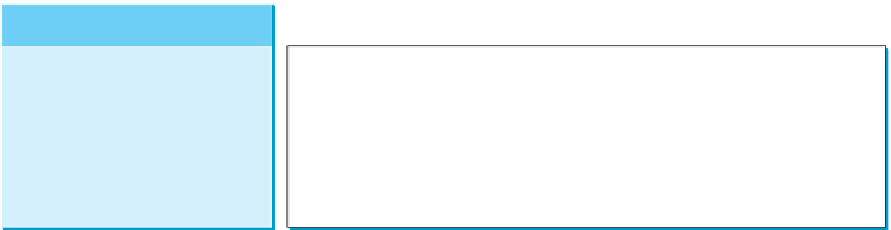


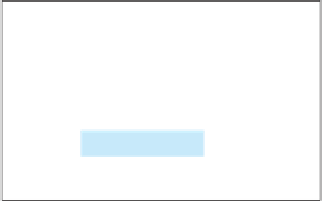


















Search WWH ::

Custom Search