Java Reference
In-Depth Information
16
/** Push a new integer to the top of the stack */
17
public void
push(
int
value) {
18
if
(size >= elements.length) {
19
int
[] temp =
new int
[elements.length *
2
];
20 System.arraycopy(elements,
0
, temp,
0
, elements.length);
21 elements = temp;
22 }
23
24 elements[size++] = value;
25 }
26
27
double the capacity
add to stack
/** Return and remove the top element from the stack */
28
public int
pop() {
29
return
elements[——size];
30 }
31
32
/** Return the top element from the stack */
33
public int
peek() {
34
return
elements[size -
1
];
35 }
36
37
/** Test whether the stack is empty */
38
public boolean
empty() {
39
return
size ==
0
;
40 }
41
42
/** Return the number of elements in the stack */
43
public int
getSize() {
44
return
size;
45 }
46 }
A primitive type value is not an object, but it can be wrapped in an object using a
wrapper class in the Java API.
Key
Point
Owing to performance considerations, primitive data type values are not objects in Java.
Because of the overhead of processing objects, the language's performance would be
adversely affected if primitive data type values were treated as objects. However, many Java
methods require the use of objects as arguments. Java offers a convenient way to incorporate,
or wrap, a primitive data type into an object (e.g., wrapping
int
into the
Integer
class,
wrapping
double
into the
Double
class, and wrapping
char
into the
Character
class,). By
using a wrapper class, you can process primitive data type values as objects. Java provides
Boolean
,
Character
,
Double
,
Float
,
Byte
,
Short
,
Integer
, and
Long
wrapper classes
in the
java.lang
package for primitive data types. The
Boolean
class wraps a Boolean
value
true
or
false
. This section uses
Integer
and
Double
as examples to introduce the
numeric wrapper classes.
why wrapper class?
Note
Most wrapper class names for a primitive type are the same as the primitive data type
name with the first letter capitalized. The exceptions are
Integer
and
Character
.
naming convention
Numeric wrapper classes are very similar to each other. Each contains the methods
doubleValue()
,
floatValue()
,
intValue()
,
longValue()
,
shortValue()
, and
byteValue()
. These methods “convert” objects into primitive type values. The key features
of
Integer
and
Double
are shown in Figure 10.14.



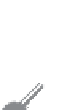


















Search WWH ::

Custom Search