Java Reference
In-Depth Information
A single array variable can reference a large collection of data.
Key
Point
Often you will have to store a large number of values during the execution of a program.
Suppose, for instance, that you need to read 100 numbers, compute their average, and find
out how many numbers are above the average. Your program first reads the numbers and
computes their average, then compares each number with the average to determine whether
it is above the average. In order to accomplish this task, the numbers must all be stored in
variables. You have to declare 100 variables and repeatedly write almost identical code
100 times. Writing a program this way would be impractical. So, how do you solve this
problem?
An efficient, organized approach is needed. Java and most other high-level languages pro-
vide a data structure, the
array
, which stores a fixed-size sequential collection of elements of
the same type. In the present case, you can store all 100 numbers into an array and access them
through a single array variable.
This chapter introduces single-dimensional arrays. The next chapter will introduce two-
dimensional and multidimensional arrays.
problem
why array?
Once an array is created, its size is fixed. An array reference variable is used to
access the elements in an array using an
index
.
Key
Point
An array is used to store a collection of data, but often we find it more useful to think of an
array as a collection of variables of the same type. Instead of declaring individual variables,
such as
number0
,
number1
, . . . , and
number99
, you declare one array variable such as
numbers
and use
numbers[0]
,
numbers[1]
, . . . , and
numbers[99]
to represent indi-
vidual variables. This section introduces how to declare array variables, create arrays, and
process arrays using indexes.
index
7.2.1 Declaring Array Variables
To use an array in a program, you must declare a variable to reference the array and specify
the array's
element type
. Here is the syntax for declaring an array variable:
element type
elementType[] arrayRefVar;
The
elementType
can be any data type, and all elements in the array will have the same
data type. For example, the following code declares a variable
myList
that references an
array of double elements.
double
[] myList;
Note
You can also use
elementType arrayRefVar[]
to declare an array variable. This
style comes from the C/C++ language and was adopted in Java to accommodate C/C++
programmers. The style
elementType[] arrayRefVar
is preferred.
preferred syntax
7.2.2 Creating Arrays
Unlike declarations for primitive data type variables, the declaration of an array variable does
not allocate any space in memory for the array. It creates only a storage location for the refer-
ence to an array. If a variable does not contain a reference to an array, the value of the variable
is
null
. You cannot assign elements to an array unless it has already been created. After an
null







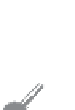




















Search WWH ::

Custom Search