Java Reference
In-Depth Information
3.26
Write a Boolean expression that evaluates to
true
if
weight
is greater than
50
pounds or height is greater than
60
inches.
3.27
Write a Boolean expression that evaluates to
true
if
weight
is greater than
50
pounds and height is greater than
60
inches.
3.28
Write a Boolean expression that evaluates to
true
if either
weight
is greater than
50
pounds or height is greater than
60
inches, but not both.
A year is a leap year if it is divisible by
4
but not by
100
, or if it is divisible by
400
.
Key
Point
You can use the following Boolean expressions to check whether a year is a leap year:
// A leap year is divisible by 4
boolean
isLeapYear = (year %
4
==
0
);
// A leap year is divisible by 4 but not by 100
isLeapYear = isLeapYear && (year %
100
!=
0
);
// A leap year is divisible by 4 but not by 100 or divisible by 400
isLeapYear = isLeapYear || (year %
400
==
0
);
Or you can combine all these expressions into one like this:
isLeapYear = (year %
4
==
0
&& year %
100
!=
0
) || (year %
400
==
0
);
Listing 3.7 gives the program that lets the user enter a year and checks whether it is a leap
year.
L
ISTING
3.7
LeapYear.java
1
import
java.util.Scanner;
2
3
public class
LeapYear {
4
public static void
main(String[] args) {
5
// Create a Scanner
6 Scanner input =
new
Scanner(System.in);
7 System.out.print(
"Enter a year: "
);
8
int
year = input.nextInt();
input
9
10
// Check if the year is a leap year
11
boolean
isLeapYear =
leap year?
12
(year %
4
==
0
&& year %
100
!=
0
) || (year %
400
==
0
);
13
14
// Display the result
15 System.out.println(year +
" is a leap year? "
+ isLeapYear);
16 }
17 }
display result
Enter a year: 2008
2008 is a leap year? true
Enter a year: 1900
1900 is a leap year? false











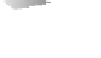







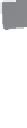
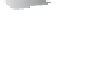




























Search WWH ::

Custom Search