Java Reference
In-Depth Information
72
/** Run a thread */
73
public void
run() {
74
try
{
75
// Create data input and output streams
76 DataInputStream inputFromClient =
new
DataInputStream(
77 socket.getInputStream());
78 DataOutputStream outputToClient =
new
DataOutputStream(
79 socket.getOutputStream());
80
81
I/O
// Continuously serve the client
82
while
(
true
) {
83
// Receive radius from the client
84
double
radius = inputFromClient.readDouble();
85
86
// Compute area
87
double
area = radius * radius * Math.PI;
88
89
// Send area back to the client
90
outputToClient.writeDouble(area);
91
92 Platform.runLater(() -> {
93 ta.appendText(
"radius received from client: "
+
94 radius +
'\n'
);
95 ta.appendText(
"Area found: "
+ area +
'\n'
);
96 });
97 }
98 }
99
catch
(IOException e) {
100 ex.printStackTrace();
101 }
102 }
103 }
104 }
update GUI
The server creates a server socket at port 8000 (line 29) and waits for a connection (line 35).
When a connection with a client is established, the server creates a new thread to handle
the communication (line 54). It then waits for another connection in an infinite
while
loop
(lines 33-55).
The threads, which run independently of one another, communicate with designated
clients. Each thread creates data input and output streams that receive and send data to a
client.
31.8
✓
✓
How do you make a server serve multiple clients?
Check
Point
A program can send and receive objects from another program.
Key
Point
In the preceding examples, you learned how to send and receive data of primitive types. You
can also send and receive objects using
ObjectOutputStream
and
ObjectInputStream
on socket streams. To enable passing, the objects must be serializable. The following example
demonstrates how to send and receive objects.
The example consists of three classes: StudentAddress.java (Listing 31.5), StudentClient.
java (Listing 31.6), and StudentServer.java (Listing 31.7). The client program collects student
information from the client and sends it to a server, as shown in Figure 31.10.
The
StudentAddress
class contains the student information: name, street, city, state,
and zip. The
StudentAddress
class implements the
Serializable
interface. Therefore, a
StudentAddress
object can be sent and received using the object output and input streams.








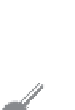
















Search WWH ::

Custom Search