Java Reference
In-Depth Information
«interface»
java.util.concurrent.Condition
+
await(): void
+
signal(): void
+
signalAll(): Condition
Causes the current thread to wait until the condition is signaled.
Wakes up one waiting thread.
Wakes up all waiting threads.
F
IGURE
30.14
The
Condition
interface defines the methods for performing
synchronization.
Let us use an example to demonstrate thread communications. Suppose that you create
and launch two tasks: one that deposits into an account and one that withdraws from the same
account. The withdraw task has to wait if the amount to be withdrawn is more than the cur-
rent balance. Whenever new funds are deposited into the account, the deposit task notifies the
withdraw thread to resume. If the amount is still not enough for a withdrawal, the withdraw
thread has to continue to wait for a new deposit.
To synchronize the operations, use a lock with a condition:
newDeposit
(i.e., new deposit
added to the account). If the balance is less than the amount to be withdrawn, the withdraw task
will wait for the
newDeposit
condition. When the deposit task adds money to the account,
the task signals the waiting withdraw task to try again. The interaction between the two tasks
is shown in Figure 30.15.
thread cooperation example
Deposit Task
Withdraw Task
lock.lock();
lock.lock();
while (balance < withdrawAmount)
newDeposit.await();
balance += depositAmount
newDeposit.signalAll();
balance -= withdrawAmount
lock.unlock();
lock.unlock();
F
IGURE
30.15
The condition
newDeposit
is used for communications between the two
threads.
You create a condition from a
Lock
object. To use a condition, you have to first obtain a
lock. The
await()
method causes the thread to wait and automatically releases the lock on the
condition. Once the condition is right, the thread reacquires the lock and continues executing.
Assume that the initial balance is
0
and the amount to deposit and withdraw are ran-
domly generated. Listing 30.6 gives the program. A sample run of the program is shown in
Figure 30.16.
F
IGURE
30.16
The withdraw task waits if there are not sufficient funds to withdraw.
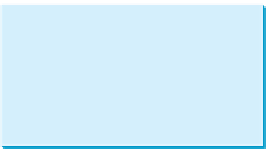







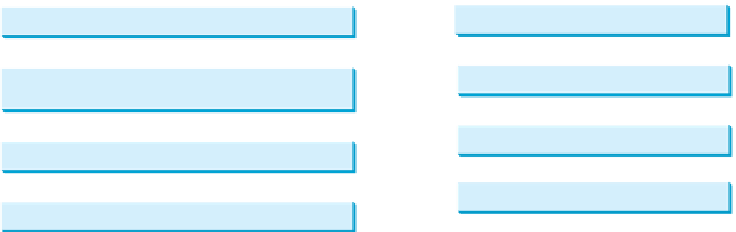



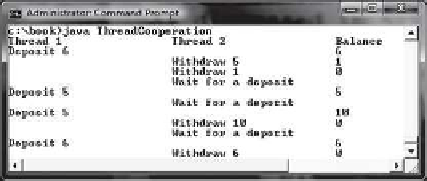
























Search WWH ::

Custom Search