Java Reference
In-Depth Information
3.13
Are the following statements correct? Which one is better?
if
(age <
16
)
System.out.println
(
"Cannot get a driver's license"
);
if
(age >=
16
)
System.out.println
(
"Can get a driver's license"
);
if
(age <
16
)
System.out.println
(
"Cannot get a driver's license"
);
else
System.out.println
(
"Can get a driver's license"
);
(a)
(b)
3.14
What is the output of the following code if
number
is
14
,
15
, or
30
?
if
(number % 2 ==
0
)
System.out.println
(number +
" is even"
);
if
(number % 5 ==
0
)
System.out.println
(number +
" is multiple of 5"
);
if
(number % 2 ==
0
)
System.out.println
(number +
" is even"
);
else if
(number % 5 ==
0
)
System.out.println
(number +
" is multiple of 5"
);
(a)
(b)
You can use
Math.random()
to obtain a random double value between
0.0
and
1.0
,
excluding
1.0
.
Key
Point
Suppose you want to develop a program for a first-grader to practice subtraction. The program
randomly generates two single-digit integers,
number1
and
number2
, with
number1 >=
number2
, and it displays to the student a question such as “What is 9
VideoNote
-
2?” After the student
enters the answer, the program displays a message indicating whether it is correct.
The previous programs generate random numbers using
System.currentTimeMillis()
.
A better approach is to use the
random()
method in the
Math
class. Invoking this method
returns a random double value
d
such that 0.0
Program subtraction quiz
random()
method
1.0. Thus,
(int)(Math.random() *
10)
returns a random single-digit integer (i.e., a number between
0
and
9
).
The program can work as follows:
…
d
6
1. Generate two single-digit integers into
number1
and
number2
.
2. If
number1 < number2
, swap
number1
with
number2
.
3. Prompt the student to answer,
"What is number1 - number2?"
4. Check the student's answer and display whether the answer is correct.
The complete program is shown in Listing 3.3.
L
ISTING
3.3
SubtractionQuiz.java
1
import
java.util.Scanner;
2
3
public class
SubtractionQuiz {
4
public static void
main(String[] args) {
5
// 1. Generate two random single-digit integers
6
int
number1 = (
int
)(Math.random() *
10
);
random number
7
int
number2 = (
int
)(Math.random() *
10
);
8
9
// 2. If number1 < number2, swap number1 with number2
10
if
(number1 < number2) {
11
int
temp = number1;
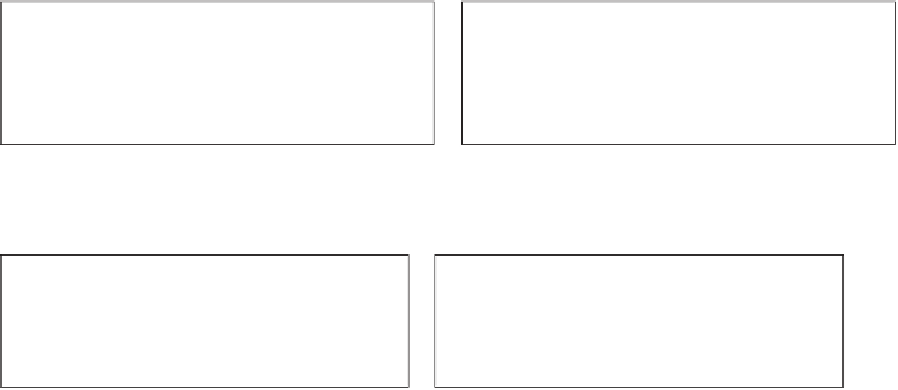



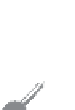






















Search WWH ::

Custom Search