Java Reference
In-Depth Information
(a) Circles are connected
(b) Circles are not connected
F
IGURE
28.14
You can apply DFS to determine whether the circles are connected.
We will write a program that lets the user create a circle by clicking a mouse in a blank
area that is not currently covered by a circle. As the circles are added, the circles are repainted
filled if they are connected or unfilled otherwise.
We will create a graph to model the problem. Each circle is a vertex in the graph. Two
circles are connected if they overlap. We apply the DFS in the graph, and if all vertices are
found in the depth-first search, the graph is connected.
The program is given in Listing 28.10.
L
ISTING
28.10
ConnectedCircles.java
1
import
javafx.application.Application;
2
import
javafx.geometry.Point2D;
3
import
javafx.scene.Node;
4
import
javafx.scene.Scene;
5
import
javafx.scene.layout.Pane;
6
import
javafx.scene.paint.Color;
7
import
javafx.scene.shape.Circle;
8
import
javafx.stage.Stage;
9
10
public class
ConnectedCircles
extends
Application {
11 @Override
// Override the start method in the Application class
12
public void
start(Stage primaryStage) {
13
// Create a scene and place it in the stage
14 Scene scene =
new
Scene(
new
CirclePane(),
450
,
350
);
15 primaryStage.setTitle(
"ConnectedCircles"
);
// Set the stage title
16 primaryStage.setScene(scene);
// Place the scene in the stage
17 primaryStage.show();
// Display the stage
18 }
19
20
/** Pane for displaying circles */
21
class
CirclePane
extends
Pane {
22
public
CirclePane() {
23
this
.setOnMouseClicked(e -> {
24
if
(!isInsideACircle(
new
Point2D(e.getX(), e.getY()))) {
25
// Add a new circle
26 getChildren().add(
new
Circle(e.getX(), e.getY(),
20
));
27 colorIfConnected();
28 }
29 });
30 }
create a circle pane
pane for showing circles
handle mouse clicked
is it inside another circle?
add a new circle
color if all connected
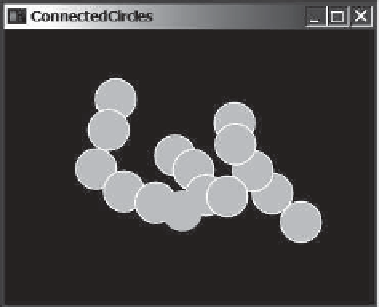
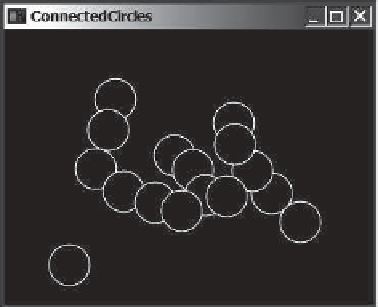















Search WWH ::

Custom Search