Game Development Reference
In-Depth Information
Before we do any of that, though, we need to load the right amount of data from the
stream. As you might have guessed, this needs to happen asynchronously, using the
LoadAsync()
method, as shown in the following code snippet:
auto loadTask = concurrency::task<unsigned
int>(reader->LoadAsync(sizeof(int)));
loadTask.then([this]
(concurrency::task<unsigned int> result)
{
if (result.get() == sizeof(int))
{
dataFromStream = reader->ReadInt32();
}
});
The result of the
LoadAsync()
method is the actual number of bytes read, which
allows you to check if you have the right amount of data, or only some of it. In the
case of the stream sockets, you may want to keep loading until you have all of the
required bytes, as any call to a Read function will throw an exception if there isn't
enough data in the reader.
Assuming everything is good, you can retrieve the data using the appropriate Read
function and continue from there.
Note
You can load in a large block of data and then read parts of it out in sequence
if desired. This would be the optimal way of doing so; however, you will need to
adjust the behavior based on what you're actually reading in and how you have
constructed the data on the other end of the connection.
The DataWriter
The counterpart to the
DataReader
is the
DataWriter
. This class handles con-
verting your data into bytes and writing them to the stream. In most cases you'll cre-
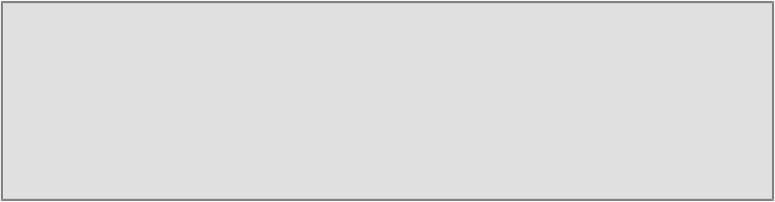